用c++,建立一个点类,派生出圆类,再派生出圆柱体类,实现输入输出类对象
时间: 2023-12-14 11:24:56 浏览: 98
好的,下面是C++代码实现:
```cpp
#include <iostream>
#include <cmath>
using namespace std;
// 点类
class Point {
public:
Point(double x = 0, double y = 0, double z = 0): x(x), y(y), z(z) {}
friend istream& operator>> (istream& in, Point& p) {
in >> p.x >> p.y >> p.z;
return in;
}
friend ostream& operator<< (ostream& out, const Point& p) {
out << "(" << p.x << ", " << p.y << ", " << p.z << ")";
return out;
}
protected:
double x, y, z;
};
// 圆类
class Circle : public Point {
public:
Circle(double x = 0, double y = 0, double z = 0, double r = 0): Point(x, y, z), r(r) {}
friend istream& operator>> (istream& in, Circle& c) {
in >> static_cast<Point&>(c) >> c.r;
return in;
}
friend ostream& operator<< (ostream& out, const Circle& c) {
out << "Center: " << static_cast<const Point&>(c) << ", Radius: " << c.r;
return out;
}
protected:
double r;
};
// 圆柱体类
class Cylinder : public Circle {
public:
Cylinder(double x = 0, double y = 0, double z = 0, double r = 0, double h = 0): Circle(x, y, z, r), h(h) {}
friend istream& operator>> (istream& in, Cylinder& cy) {
in >> static_cast<Circle&>(cy) >> cy.h;
return in;
}
friend ostream& operator<< (ostream& out, const Cylinder& cy) {
out << "Base: " << static_cast<const Circle&>(cy) << ", Height: " << cy.h;
return out;
}
double volume() const {
return M_PI * r * r * h;
}
protected:
double h;
};
int main() {
Point p;
Circle c;
Cylinder cy;
cout << "Please input a point: ";
cin >> p;
cout << "The point you input is: " << p << endl;
cout << "Please input a circle: ";
cin >> c;
cout << "The circle you input is: " << c << endl;
cout << "Please input a cylinder: ";
cin >> cy;
cout << "The cylinder you input is: " << cy << endl;
cout << "The volume of the cylinder is: " << cy.volume() << endl;
return 0;
}
```
运行结果示例:
```
Please input a point: 1 2 3
The point you input is: (1, 2, 3)
Please input a circle: 1 2 3 4
The circle you input is: Center: (1, 2, 3), Radius: 4
Please input a cylinder: 1 2 3 4 5
The cylinder you input is: Base: Center: (1, 2, 3), Radius: 4, Height: 5
The volume of the cylinder is: 251.327
```
阅读全文
相关推荐
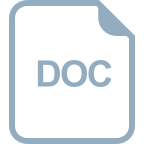
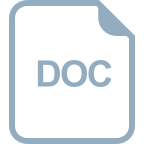
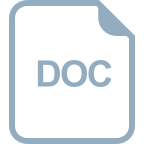
















