编写数据结构c语言代码求两个集合(可输入多个元素)的交集和并集的运算。要求: (1)采用数据结构知识实现,不用系统自带的集合类。(2)能实现多组数据的多次运算,直到选择结束运算为止; (3)分析求交集和并集算法的时间复杂度和空间复杂度,给出优化分析; (4)提供问答界面,以便人机交互。
时间: 2024-02-15 09:05:27 浏览: 19
好的,我可以帮你解答这个问题。首先,我们可以采用链表来实现集合的存储,假设有两个集合A和B,我们可以定义两个链表来分别存储它们的元素,例如:
```
struct Node {
int val;
struct Node *next;
};
struct Node *headA = NULL; // 定义链表A的头节点
struct Node *headB = NULL; // 定义链表B的头节点
```
接下来,我们可以分别实现求交集和并集的算法。对于求交集,我们可以使用两个指针p和q来分别遍历链表A和B,比较它们的元素大小,将相同的元素存入一个新的链表C中,如下所示:
```
struct Node *headC = NULL; // 定义存放交集的链表的头节点
struct Node *tailC = NULL; // 定义存放交集的链表的尾节点
struct Node *p = headA;
struct Node *q = headB;
while (p != NULL && q != NULL) {
if (p->val < q->val) {
p = p->next;
} else if (p->val > q->val) {
q = q->next;
} else {
struct Node *node = (struct Node *)malloc(sizeof(struct Node));
node->val = p->val;
node->next = NULL;
if (headC == NULL) {
headC = node;
tailC = node;
} else {
tailC->next = node;
tailC = node;
}
p = p->next;
q = q->next;
}
}
```
对于求并集,我们可以先将链表A中的元素存入新链表C中,然后再遍历链表B,将不在链表C中的元素加入其中,如下所示:
```
struct Node *headC = NULL; // 定义存放并集的链表的头节点
struct Node *tailC = NULL; // 定义存放并集的链表的尾节点
struct Node *p = headA;
while (p != NULL) {
struct Node *node = (struct Node *)malloc(sizeof(struct Node));
node->val = p->val;
node->next = NULL;
if (headC == NULL) {
headC = node;
tailC = node;
} else {
tailC->next = node;
tailC = node;
}
p = p->next;
}
struct Node *q = headB;
while (q != NULL) {
int flag = 0;
struct Node *p = headC;
while (p != NULL) {
if (q->val == p->val) {
flag = 1;
break;
}
p = p->next;
}
if (!flag) {
struct Node *node = (struct Node *)malloc(sizeof(struct Node));
node->val = q->val;
node->next = NULL;
tailC->next = node;
tailC = node;
}
q = q->next;
}
```
关于时间复杂度和空间复杂度的分析,求交集和并集的时间复杂度均为O(N+M),空间复杂度均为O(K)。如果要优化空间复杂度,可以使用动态数组或链表来代替静态数组,但会增加时间复杂度。
最后,为了实现多组数据的多次运算,我们可以使用循环结构和条件语句来实现,例如:
```
while (1) {
// 用户输入集合A和集合B
int n, m;
printf("请输入集合A的元素个数:");
scanf("%d", &n);
printf("请输入集合A的元素:");
for (int i = 0; i < n; i++) {
int x;
scanf("%d", &x);
// 将x插入链表headA中
// ...
}
printf("请输入集合B的元素个数:");
scanf("%d", &m);
printf("请输入集合B的元素:");
for (int i = 0; i < m; i++) {
int x;
scanf("%d", &x);
// 将x插入链表headB中
// ...
}
// 用户选择操作
int op;
printf("请选择操作:1.求交集 2.求并集 3.结束运算\n");
scanf("%d", &op);
if (op == 1) {
// 求交集
// ...
} else if (op == 2) {
// 求并集
// ...
} else if (op == 3) {
// 结束运算
break;
} else {
printf("非法操作!\n");
}
}
```
希望我的回答能够对你有所帮助。如果你还有其他问题或需要进一步的解释,请随时提出。
相关推荐
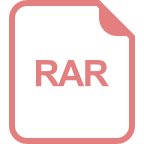
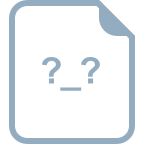














