linux 下 cpp 向串口发送命令(16进制数) 并接收串口返回数据(16进制数)
时间: 2023-08-30 14:04:25 浏览: 98
在 Linux 下,可以通过串口设备文件对串口进行读写操作。以下是一个简单的 C++ 程序示例,用于向串口发送命令并接收返回数据(假设串口设备文件为 `/dev/ttyS0`):
```cpp
#include <stdio.h>
#include <stdlib.h>
#include <fcntl.h>
#include <termios.h>
#include <unistd.h>
#include <string.h>
int main()
{
int fd;
struct termios options;
// 打开串口设备文件
fd = open("/dev/ttyS0", O_RDWR | O_NOCTTY | O_NDELAY);
if (fd == -1) {
perror("open");
exit(1);
}
// 配置串口
tcgetattr(fd, &options);
cfsetispeed(&options, B9600);
cfsetospeed(&options, B9600);
options.c_cflag |= CLOCAL | CREAD;
options.c_cflag &= ~CSIZE;
options.c_cflag |= CS8;
options.c_cflag &= ~CSTOPB;
options.c_cflag &= ~PARENB;
tcsetattr(fd, TCSANOW, &options);
// 发送命令
unsigned char buf[4] = {0x01, 0x02, 0x03, 0x04};
write(fd, buf, 4);
// 接收返回数据
unsigned char recv_buf[4] = {0};
int n = read(fd, recv_buf, 4);
if (n == -1) {
perror("read");
exit(1);
}
printf("Received %d bytes:", n);
for (int i = 0; i < n; i++) {
printf(" %02X", recv_buf[i]);
}
printf("\n");
// 关闭串口
close(fd);
return 0;
}
```
在这个程序中,我们首先打开了 `/dev/ttyS0` 设备文件,然后配置了串口参数(波特率为 9600,数据位为 8,无校验位,停止位为 1)。然后我们发送了一个长度为 4 的命令,接着通过 `read()` 函数接收了 4 个字节的返回数据,并打印出来。最后我们关闭了串口设备文件。
需要注意的是,在 Linux 下,串口设备文件的权限可能需要设置为可读写才能进行操作。如果出现权限问题,可以使用 `sudo` 命令运行程序。
阅读全文
相关推荐
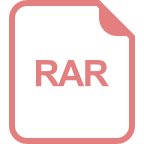
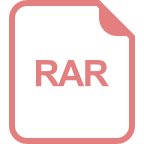

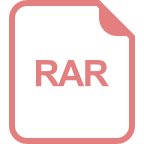













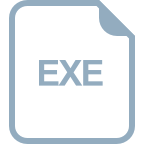