#include <stdio.h> #define MAXSIZE 100 typedef struct { int n, e; char V[MAXSIZE]; int edges[MAXSIZE][MAXSIZE]; }MGraph; void CreateMG(MGraph* G) { int i, j, k; char ch1, ch2; printf("请输入顶点数:"); scanf_s("%d", &G->n); printf("请输入边数:"); scanf_s("%d", &G->e); printf("请输入各顶点信息:"); for (i = 0; i < G->n; i++) { getchar(); printf("请输入第%d个顶点:", i + 1); scanf_s("%c", &(G->V[i])); } for (i = 0; i < G->n; i++) for (j = 0; j < G->n; j++) G->edges[i][j] = 0; for (k = 0; k < G->e; k++) { getchar(); printf("建立第%d条边:", k + 1); scanf_s("%c,%c", &ch1, &ch2); for (i = 0; i < G->n; i++) for (j = 0; j < G->n; j++) if (ch1 == G->V[i] && ch2 == G->V[j]) { G->edges[i][j] = 1; G->edges[j][i] = 1; } } } void main() { MGraph G; CreateMG(&G); }完善简化此代码
时间: 2024-01-31 10:03:04 浏览: 69
这段代码是用来创建一个无向图的邻接矩阵的。下面是代码的分析:
1. 定义一个结构体MGraph,包含了图的顶点数目n,边数e,顶点信息V,边的邻接矩阵edges。
2. 定义了一个函数CreateMG,用来创建无向图的邻接矩阵。函数中先输入顶点数和边数,然后输入各个顶点的信息,存入V数组中。接着初始化邻接矩阵的所有元素为0,然后输入每一条边的起点和终点,根据V数组中的信息建立邻接矩阵。
3. 在主函数中创建一个MGraph类型的变量G,然后调用CreateMG函数,将G的地址传入函数中,进行创建无向图的邻接矩阵。
为了完善和简化代码,我们可以进行以下改进:
1. 将输入顶点信息和建立边的操作分开,可以让代码结构更加清晰。
2. 可以将输入顶点信息的过程提取出来,作为一个独立的函数,让代码更加模块化。
3. 可以考虑使用动态内存分配,避免数组大小不足的问题。
下面是改进后的代码:
```
#include <stdio.h>
#include <stdlib.h>
typedef struct {
int n, e;
char* V;
int** edges;
} MGraph;
void CreateVertices(MGraph* G) {
G->V = (char*)malloc(G->n * sizeof(char));
if (G->V == NULL) {
printf("Error: memory allocation failed!\n");
exit(1);
}
printf("请输入各顶点信息:");
for (int i = 0; i < G->n; i++) {
getchar();
printf("请输入第%d个顶点:", i + 1);
scanf("%c", &(G->V[i]));
}
}
void CreateEdges(MGraph* G) {
G->edges = (int**)malloc(G->n * sizeof(int*));
if (G->edges == NULL) {
printf("Error: memory allocation failed!\n");
exit(1);
}
for (int i = 0; i < G->n; i++) {
G->edges[i] = (int*)calloc(G->n, sizeof(int));
if (G->edges[i] == NULL) {
printf("Error: memory allocation failed!\n");
exit(1);
}
}
char ch1, ch2;
for (int k = 0; k < G->e; k++) {
getchar();
printf("建立第%d条边:", k + 1);
scanf("%c,%c", &ch1, &ch2);
int i, j;
for (i = 0; i < G->n; i++) {
if (ch1 == G->V[i]) break;
}
for (j = 0; j < G->n; j++) {
if (ch2 == G->V[j]) break;
}
if (i == G->n || j == G->n) {
printf("Error: invalid edge!\n");
exit(1);
}
G->edges[i][j] = 1;
G->edges[j][i] = 1;
}
}
void CreateMG(MGraph* G) {
printf("请输入顶点数:");
scanf("%d", &G->n);
printf("请输入边数:");
scanf("%d", &G->e);
CreateVertices(G);
CreateEdges(G);
}
int main() {
MGraph G;
CreateMG(&G);
return 0;
}
```
阅读全文
相关推荐
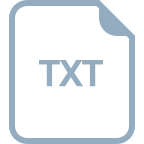
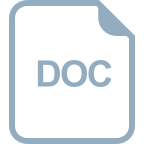
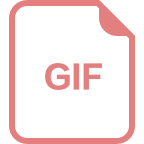
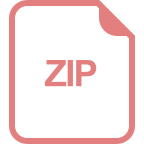













