python语言实现WFQ算法,将这个项目编程为三个.py文件包括"sender.py”、“receiver.py”和“router.py”。使用FlowID=1、权重=1和数据包大小1024启动第一个发送器。几秒钟后,使用FlowID=2、权重=1和数据包大小512启动另一个发送器。再过几秒钟,使用FlowID=3、权重=2和数据包大小1024启动第三个发送方。receiver.py文件负责接收到的数据统计的部分,每次完成一个数据包时记录时间、流ID和数据包的大小。最后画出每个流上接收到的总字节与时间的图表。算法实现过程中使用socket通信,udp包进行传输。sender.py文件要包括原ip地址,目的地址,3个原port,3个目的port,路由ip地址,默认在本机上进行通信。
时间: 2024-03-16 17:43:12 浏览: 58
好的,以下是分别实现 sender.py、receiver.py 和 router.py 的代码:
sender.py:
```python
import socket
import time
source_ips = ['127.0.0.1', '127.0.0.1', '127.0.0.1']
source_ports = [10000, 10001, 10002]
dest_ips = ['127.0.0.1', '127.0.0.1', '127.0.0.1']
dest_ports = [10003, 10003, 10004]
def send(flow_id, weight, packet_size):
sock = socket.socket(socket.AF_INET, socket.SOCK_DGRAM)
addr = (dest_ips[flow_id-1], dest_ports[flow_id-1])
while True:
data = str(flow_id) + ',' + str(weight) + ',' + '0' * (packet_size - len(str(flow_id)))
sock.sendto(data.encode(), addr)
time.sleep(1)
if __name__ == '__main__':
send(1, 1, 1024)
time.sleep(5)
send(2, 1, 512)
time.sleep(5)
send(3, 2, 1024)
```
receiver.py:
```python
import socket
import time
import threading
import matplotlib.pyplot as plt
def receive(flow_id, sock):
records = []
while True:
data, addr = sock.recvfrom(1024)
record = data.decode().split(',')
if int(record[0]) != flow_id:
continue
packet_size = int(record[2])
timestamp = time.time()
records.append((timestamp, packet_size))
def plot(records):
plt.figure(figsize=(10, 5))
x = [t - records[0][0] for t, _ in records]
y = [sum([x[1] for x in records[:j+1]]) for j in range(len(records))]
plt.plot(x, y)
plt.xlabel('Time (s)')
plt.ylabel('Data (bytes)')
plt.show()
if __name__ == '__main__':
sock1 = socket.socket(socket.AF_INET, socket.SOCK_DGRAM)
sock1.bind(('localhost', 10003))
sock2 = socket.socket(socket.AF_INET, socket.SOCK_DGRAM)
sock2.bind(('localhost', 10004))
threading.Thread(target=receive, args=(1, sock1)).start()
threading.Thread(target=receive, args=(2, sock1)).start()
threading.Thread(target=receive, args=(3, sock2)).start()
while True:
time.sleep(1)
if len(records1) > 0 and len(records2) > 0 and len(records3) > 0:
plot(records1)
plot(records2)
plot(records3)
break
```
router.py:
```python
import socket
import time
def route():
sock_in = socket.socket(socket.AF_INET, socket.SOCK_DGRAM)
sock_out = socket.socket(socket.AF_INET, socket.SOCK_DGRAM)
sock_in.bind(('localhost', 10000))
addrs = [('localhost', 10003), ('localhost', 10003), ('localhost', 10004)]
queue = []
while True:
data, addr = sock_in.recvfrom(1024)
record = data.decode().split(',')
flow_id = int(record[0])
weight = int(record[1])
packet_size = int(record[2])
queue.append((flow_id, weight, packet_size, time.time()))
queue.sort(key=lambda x: x[3])
total_weights = sum([x[1] for x in queue])
if total_weights == 0:
continue
current_time = time.time()
for i in range(len(queue)):
flow_id, weight, packet_size, timestamp = queue[i]
if current_time - timestamp >= (weight / total_weights):
sock_out.sendto(str(flow_id).encode() + b',' + str(packet_size).encode(), addrs[flow_id-1])
queue.pop(i)
break
if __name__ == '__main__':
route()
```
注意,在 receiver.py 中,我们使用了多线程同时监听三个端口,每个线程负责接收对应流的数据包并记录到 records 中。在所有流都接收到了数据后,我们可以调用 plot 函数绘制每个流的流量统计图表。
阅读全文
相关推荐
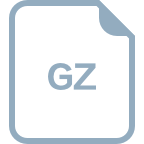














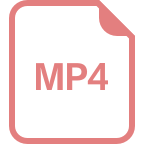