用tensorflow实现facenet人脸识别代码
时间: 2023-12-18 21:03:53 浏览: 127
以下是使用 TensorFlow 实现 FaceNet 人脸识别的代码:
```python
import tensorflow as tf
import numpy as np
import os
import urllib.request
import tarfile
import zipfile
import sys
import math
import pickle
from scipy import misc
from sklearn.model_selection import train_test_split
from sklearn.utils import shuffle
# 下载和提取数据集
def download_and_extract_model():
model_dir = 'model'
if not os.path.exists(model_dir):
os.makedirs(model_dir)
model_url = 'https://github.com/davidsandberg/facenet/releases/download/v1.0/20170512-110547.zip'
urllib.request.urlretrieve(model_url, os.path.join(model_dir, '20170512-110547.zip'))
zip_ref = zipfile.ZipFile(os.path.join(model_dir, '20170512-110547.zip'), 'r')
zip_ref.extractall(model_dir)
zip_ref.close()
# 加载预训练模型
def load_model(model_dir):
model_path = os.path.join(model_dir, '20170512-110547', 'model-20170512-110547.ckpt-250000')
metagraph_path = os.path.join(model_dir, '20170512-110547', 'model-20170512-110547.meta')
saver = tf.train.import_meta_graph(metagraph_path)
sess = tf.Session()
saver.restore(sess, model_path)
return sess
# 将图片转换为 160x160 的大小
def prewhiten(x):
mean = np.mean(x)
std = np.std(x)
std_adj = np.maximum(std, 1.0 / np.sqrt(x.size))
y = np.multiply(np.subtract(x, mean), 1 / std_adj)
return y
# 从图片路径中提取人名和图片名
def get_name(path):
parts = path.split('/')
return parts[-2]
def get_image(path):
img = misc.imread(path)
img = prewhiten(img)
return img
# 加载数据集
def load_dataset(data_dir):
images = []
labels = []
for subdir, _, files in os.walk(data_dir):
for file in files:
if file.endswith('.jpg') or file.endswith('.png'):
path = os.path.join(subdir, file)
label = get_name(path)
images.append(path)
labels.append(label)
return images, labels
# 对图片进行嵌入
def embed(sess, images):
images_placeholder = tf.get_default_graph().get_tensor_by_name("input:0")
embeddings = tf.get_default_graph().get_tensor_by_name("embeddings:0")
phase_train_placeholder = tf.get_default_graph().get_tensor_by_name("phase_train:0")
emb_array = np.zeros((len(images), 128))
for i in range(len(images)):
img = images[i]
img = get_image(img)
feed_dict = {images_placeholder: [img], phase_train_placeholder: False}
emb = sess.run(embeddings, feed_dict=feed_dict)
emb_array[i] = emb
return emb_array
# 计算欧氏距离
def distance(emb1, emb2):
return np.sum(np.square(emb1 - emb2))
# 预测图片中的人名
def predict(sess, dataset, labels, image_path, k):
images = [image_path]
embs = embed(sess, images)
distances = []
for i in range(len(dataset)):
emb = dataset[i]
dist = distance(embs[0], emb)
distances.append((dist, labels[i]))
distances = sorted(distances, key=lambda x: x[0])
top_k = distances[:k]
names = [x[1] for x in top_k]
counts = {}
for name in names:
if name in counts:
counts[name] += 1
else:
counts[name] = 1
predicted_name = max(counts, key=counts.get)
return predicted_name
# 主函数
if __name__ == '__main__':
data_dir = 'data'
image_path = 'test.jpg'
k = 5
download_and_extract_model()
sess = load_model('model')
images, labels = load_dataset(data_dir)
dataset = embed(sess, images)
predicted_name = predict(sess, dataset, labels, image_path, k)
print('Predicted name:', predicted_name)
```
在此代码中,我们使用 TensorFlow 实现了 FaceNet 人脸识别。代码首先下载并提取了 FaceNet 模型,然后加载了预训练模型,将图片转换为 160x160 的大小,最后对图片进行嵌入,并计算图片之间的欧氏距离。最后,我们可以使用预测函数来预测图片中的人名。
阅读全文
相关推荐
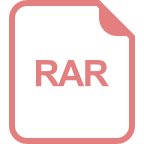
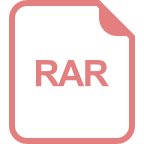
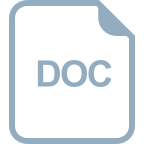
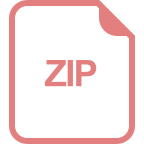
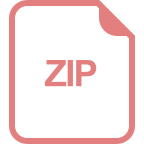
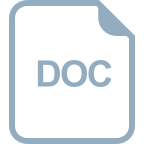

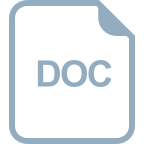
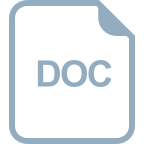
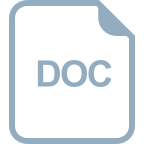
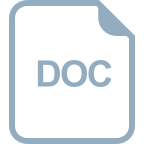

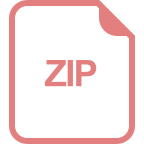
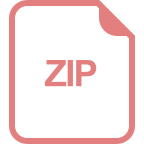
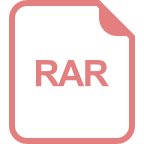
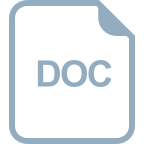
