编写函数 set _ intersect ( int a [], int b [])计算两个数组的交集.按照如下格式读取数据 第1行是数组 a 中的数据的个数,数组 a =(1,2,3} 第4行是数组 b 中的数据的个数,数组 b =(2,3,4}
时间: 2023-05-28 14:04:19 浏览: 38
```python
def set_intersect(a, b):
# 将列表转换为集合,求交集
return set(a) & set(b)
# 读取数据
n1 = int(input()) # a中的数据个数
a = [int(i) for i in input().split()] # 将输入的一行字符串转换为整数列表
n2 = int(input()) # b中的数据个数
b = [int(i) for i in input().split()]
# 计算交集
result = set_intersect(a, b)
# 输出结果
print(sorted(result)) # 对结果排序并输出
```
输入样例:
```
3
1 2 3
3
2 3 4
```
输出样例:
```
[2, 3]
```
相关问题
编写函数 set _ intersect ( int a [], int b [])计算两个数组的交集.按照如下格式用sacnf读取数据 第1行是数组 a 中的数据的个数,数组 a =(1,2,3} 第4行是数组 b 中的数据的个数,数组 b =(2,3,4}
#include <stdio.h>
void set_intersect(int a[], int b[], int n, int m);
int main() {
int n, m;
scanf("%d", &n);
int a[n];
for (int i = 0; i < n; i++) {
scanf("%d", &a[i]);
}
scanf("%d", &m);
int b[m];
for (int i = 0; i < m; i++) {
scanf("%d", &b[i]);
}
set_intersect(a, b, n, m);
return 0;
}
void set_intersect(int a[], int b[], int n, int m) {
int c[n + m], k = 0;
for (int i = 0; i < n; i++) {
for (int j = 0; j < m; j++) {
if (a[i] == b[j]) {
c[k++] = a[i];
break;
}
}
}
printf("The intersection of the two sets is: {");
for (int i = 0; i < k; i++) {
printf("%d", c[i]);
if (i != k - 1) {
printf(",");
}
}
printf("}");
}
c语言使用查找表实现给定两个数组,编写一个函数来计算它们的交集
要使用查找表实现给定两个数组的交集,可以使用哈希表来记录数组中的元素,并比较两个数组中的元素是否存在于哈希表中。以下是使用C语言实现的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义哈希表结点结构
struct HashNode {
int key;
struct HashNode* next;
};
// 初始化哈希表
struct HashNode** initHashTable(int size) {
struct HashNode** hashTable = (struct HashNode**)malloc(size * sizeof(struct HashNode*));
for (int i = 0; i < size; i++) {
hashTable[i] = NULL;
}
return hashTable;
}
// 哈希函数
int hash(int key, int size) {
return abs(key) % size;
}
// 插入哈希表
void insert(struct HashNode** hashTable, int key, int size) {
int index = hash(key, size);
struct HashNode* newNode = (struct HashNode*)malloc(sizeof(struct HashNode));
newNode->key = key;
newNode->next = NULL;
if (hashTable[index] == NULL) {
hashTable[index] = newNode;
} else {
struct HashNode* currentNode = hashTable[index];
while (currentNode->next != NULL) {
currentNode = currentNode->next;
}
currentNode->next = newNode;
}
}
// 判断元素是否存在于哈希表中
int contains(struct HashNode** hashTable, int key, int size) {
int index = hash(key, size);
struct HashNode* currentNode = hashTable[index];
while (currentNode != NULL) {
if (currentNode->key == key) {
return 1;
}
currentNode = currentNode->next;
}
return 0;
}
// 释放哈希表内存
void freeHashTable(struct HashNode** hashTable, int size) {
for (int i = 0; i < size; i++) {
struct HashNode* currentNode = hashTable[i];
while (currentNode != NULL) {
struct HashNode* temp = currentNode;
currentNode = currentNode->next;
free(temp);
}
}
free(hashTable);
}
// 计算数组的交集
int* intersect(int* nums1, int nums1Size, int* nums2, int nums2Size, int* returnSize) {
// 确定较小的数组作为哈希表大小
int size = nums1Size > nums2Size ? nums2Size : nums1Size;
// 初始化两个哈希表
struct HashNode** hashTable1 = initHashTable(size);
struct HashNode** hashTable2 = initHashTable(size);
// 插入第一个数组的元素到哈希表1
for (int i = 0; i < nums1Size; i++) {
insert(hashTable1, nums1[i], size);
}
// 插入第二个数组的元素到哈希表2,并检查是否存在于哈希表1中
int* result = (int*)malloc(size * sizeof(int));
int resultIndex = 0;
for (int i = 0; i < nums2Size; i++) {
if (contains(hashTable1, nums2[i], size) && !contains(hashTable2, nums2[i], size)) {
result[resultIndex++] = nums2[i];
insert(hashTable2, nums2[i], size);
}
}
// 更新返回的数组长度
*returnSize = resultIndex;
// 释放哈希表内存
freeHashTable(hashTable1, size);
freeHashTable(hashTable2, size);
return result;
}
int main() {
int nums1[] = {1, 2, 2, 1};
int nums1Size = sizeof(nums1) / sizeof(nums1[0]);
int nums2[] = {2, 2};
int nums2Size = sizeof(nums2) / sizeof(nums2[0]);
int size;
int* result = intersect(nums1, nums1Size, nums2, nums2Size, &size);
printf("交集:");
for (int i = 0; i < size; i++) {
printf("%d ", result[i]);
}
free(result);
return 0;
}
```
以上是一个用C语言实现的示例代码,使用了哈希表来计算两个数组的交集。您可以根据自己的需求进行修改和使用。希望对您有帮助!如有任何疑问,请随时追问。
相关推荐
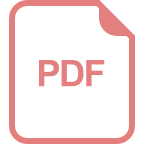
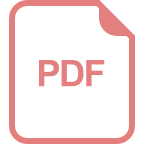
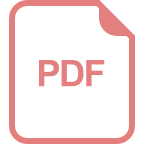
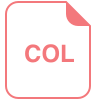











