用c#写一个飞机大战的游戏
时间: 2023-12-02 11:06:32 浏览: 35
好的,以下是一个简单的飞机大战游戏的 C# 代码示例:
```csharp
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Drawing;
using System.Windows.Forms;
namespace PlaneGame
{
class Program : Form
{
private int planeX = 100;
private int planeY = 300;
private int enemyX = 700;
private int enemyY = 150;
private int score = 0;
private Timer timer;
private Random random;
private Font font;
private Pen pen;
private Brush brush;
private Image planeImage;
private Image enemyImage;
private Image backgroundImage;
public Program()
{
Text = "Plane Game";
Width = 800;
Height = 600;
timer = new Timer();
timer.Interval = 20;
timer.Tick += Timer_Tick;
timer.Start();
random = new Random();
font = new Font("Arial", 16);
pen = new Pen(Color.Red);
brush = new SolidBrush(Color.White);
planeImage = Image.FromFile(@"plane.png");
enemyImage = Image.FromFile(@"enemy.png");
backgroundImage = Image.FromFile(@"background.png");
DoubleBuffered = true;
}
private void Timer_Tick(object sender, EventArgs e)
{
// move enemy
enemyX -= 5;
if (enemyX < -100) // enemy is off screen
{
enemyX = 800;
enemyY = random.Next(100, 400);
score++; // increment score
}
// check collision
Rectangle planeRect = new Rectangle(planeX, planeY, 100, 50);
Rectangle enemyRect = new Rectangle(enemyX, enemyY, 100, 50);
if (planeRect.IntersectsWith(enemyRect))
{
timer.Stop(); // game over
MessageBox.Show("Game Over! Score: " + score.ToString());
Close();
}
// redraw
Invalidate();
}
protected override void OnPaint(PaintEventArgs e)
{
base.OnPaint(e);
// draw background
e.Graphics.DrawImage(backgroundImage, 0, 0);
// draw plane
e.Graphics.DrawImage(planeImage, planeX, planeY);
// draw enemy
e.Graphics.DrawImage(enemyImage, enemyX, enemyY);
// draw score
e.Graphics.DrawString("Score: " + score.ToString(), font, brush, 10, 10);
}
protected override void OnKeyDown(KeyEventArgs e)
{
base.OnKeyDown(e);
// move plane
if (e.KeyCode == Keys.Up && planeY > 0)
{
planeY -= 10;
}
else if (e.KeyCode == Keys.Down && planeY < 550)
{
planeY += 10;
}
else if (e.KeyCode == Keys.Space) // shoot
{
// TODO: implement shooting
}
}
static void Main(string[] args)
{
Application.Run(new Program());
}
}
}
```
在此示例中,我们使用了 Windows 窗体和 GDI+ 绘图功能来实现游戏的界面和动画。在游戏中,玩家控制飞机移动,并尝试躲避敌机,同时可以按下空格键进行射击。游戏会根据时间和碰撞检测来更新游戏状态,并随时重绘界面。当玩家撞上敌机时,游戏结束并弹出得分对话框。
请注意,此示例只是一个简单的演示,还有许多改进和扩展可以进行。例如,您可以添加更多的敌机、障碍物和武器,改进碰撞检测算法,增加音效和动画效果等等。祝您好运!
相关推荐
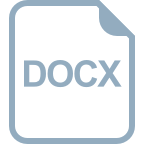














