用c++写一个代码实现常见的链表操作,插入头部,尾部,某数据之后或之前,修改,删除,排序,存储到文件,从文件构建链表,判空,是否存在,清空,排序,
时间: 2023-05-31 17:03:09 浏览: 120
以下是用C语言实现常见的链表操作的代码:
```
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构体
typedef struct Node {
int data;
struct Node *next;
} Node;
// 定义链表结构体
typedef struct List {
Node *head;
Node *tail;
} List;
// 初始化链表
List *initList() {
List *list = (List *)malloc(sizeof(List));
Node *head = (Node *)malloc(sizeof(Node));
head->next = NULL;
list->head = head;
list->tail = head;
return list;
}
// 判断链表是否为空
int isEmpty(List *list) {
return list->head->next == NULL;
}
// 在链表头部插入节点
void insertHead(List *list, int data) {
Node *node = (Node *)malloc(sizeof(Node));
node->data = data;
node->next = list->head->next;
list->head->next = node;
if (list->tail == list->head) {
list->tail = node;
}
}
// 在链表尾部插入节点
void insertTail(List *list, int data) {
Node *node = (Node *)malloc(sizeof(Node));
node->data = data;
node->next = NULL;
list->tail->next = node;
list->tail = node;
}
// 在某个节点之后插入节点
void insertAfter(List *list, int afterData, int data) {
Node *node = (Node *)malloc(sizeof(Node));
node->data = data;
Node *p = list->head->next;
while (p != NULL) {
if (p->data == afterData) {
node->next = p->next;
p->next = node;
if (list->tail == p) {
list->tail = node;
}
break;
}
p = p->next;
}
}
// 在某个节点之前插入节点
void insertBefore(List *list, int beforeData, int data) {
Node *node = (Node *)malloc(sizeof(Node));
node->data = data;
Node *p = list->head;
while (p->next != NULL) {
if (p->next->data == beforeData) {
node->next = p->next;
p->next = node;
if (list->tail == p) {
list->tail = node;
}
break;
}
p = p->next;
}
}
// 修改某个节点的值
void modify(List *list, int oldData, int newData) {
Node *p = list->head->next;
while (p != NULL) {
if (p->data == oldData) {
p->data = newData;
break;
}
p = p->next;
}
}
// 删除某个节点
void delete(List *list, int data) {
Node *p = list->head;
while (p->next != NULL) {
if (p->next->data == data) {
Node *temp = p->next;
p->next = temp->next;
free(temp);
if (list->tail == temp) {
list->tail = p;
}
break;
}
p = p->next;
}
}
// 排序链表(升序)
void sort(List *list) {
Node *p = list->head->next;
int len = 0;
while (p != NULL) {
len++;
p = p->next;
}
for (int i = 0; i < len - 1; i++) {
p = list->head->next;
for (int j = 0; j < len - 1 - i; j++) {
if (p->data > p->next->data) {
int temp = p->data;
p->data = p->next->data;
p->next->data = temp;
}
p = p->next;
}
}
}
// 存储链表到文件
void save(List *list, char *filename) {
FILE *fp = fopen(filename, "w");
Node *p = list->head->next;
while (p != NULL) {
fprintf(fp, "%d\n", p->data);
p = p->next;
}
fclose(fp);
}
// 从文件构建链表
List *load(char *filename) {
List *list = initList();
FILE *fp = fopen(filename, "r");
if (fp == NULL) {
return list;
}
int data;
while (fscanf(fp, "%d", &data) != EOF) {
insertTail(list, data);
}
fclose(fp);
return list;
}
// 判断某个节点是否存在
int exists(List *list, int data) {
Node *p = list->head->next;
while (p != NULL) {
if (p->data == data) {
return 1;
}
p = p->next;
}
return 0;
}
// 清空链表
void clear(List *list) {
Node *p = list->head->next;
while (p != NULL) {
Node *temp = p;
p = p->next;
free(temp);
}
list->head->next = NULL;
list->tail = list->head;
}
// 输出链表中的所有元素
void print(List *list) {
Node *p = list->head->next;
while (p != NULL) {
printf("%d ", p->data);
p = p->next;
}
printf("\n");
}
int main() {
List *list = initList();
insertHead(list, 3);
insertHead(list, 2);
insertHead(list, 1);
insertTail(list, 4);
insertAfter(list, 2, 5);
insertBefore(list, 4, 6);
modify(list, 2, 7);
delete(list, 3);
sort(list);
save(list, "list.txt");
List *newList = load("list.txt");
printf("List: ");
print(list);
printf("New List: ");
print(newList);
printf("Exists 2: %d\n", exists(list, 2));
printf("Exists 8: %d\n", exists(list, 8));
clear(list);
printf("List is empty: %d\n", isEmpty(list));
return 0;
}
```
阅读全文
相关推荐
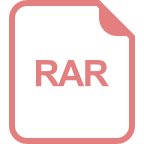















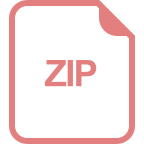

