已经转化好的onnx模型通道顺序是NHWC怎么转换成NCHW python
时间: 2024-04-01 20:36:04 浏览: 19
可以使用numpy库将NHWC格式的张量转换成NCHW格式的张量。具体的代码实现如下:
```python
import numpy as np
# 假设NHWC格式的张量为x_nhwc
x_nhwc = np.random.rand(batch_size, height, width, channel)
# 将NHWC格式的张量转换成NCHW格式的张量
x_nchw = np.transpose(x_nhwc, (0, 3, 1, 2))
```
其中,transpose函数的第一个参数为要转换的张量,第二个参数为转换后的维度顺序,本例中转换后的维度顺序为(0, 3, 1, 2),即batch_size、channel、height、width的顺序。转换后的张量x_nchw即为NCHW格式的张量。
相关问题
已经转化好的.onnx模型通道顺序是NHWC怎么转换成NCHW 的.onnx 模型 python
可以使用onnx包中的API对.onnx模型进行通道顺序的转换。具体的代码实现如下:
```python
import onnx
from onnx import numpy_helper
# 加载模型
model = onnx.load('model.onnx')
# 获取输入节点和输入张量的信息
input_name = model.graph.input[0].name
input_shape = model.graph.input[0].type.tensor_type.shape.dim
input_dtype = model.graph.input[0].type.tensor_type.elem_type
# 将输入节点的通道顺序从NHWC修改为NCHW
input_shape[1].dim_value, input_shape[2].dim_value, input_shape[3].dim_value = input_shape[3].dim_value, input_shape[1].dim_value, input_shape[2].dim_value
# 创建新的输入张量
new_input_tensor = numpy_helper.from_array(np.zeros([batch_size, channel, height, width], dtype=np.float32), input_name)
# 将新的输入张量添加到模型中
model.graph.input.remove(model.graph.input[0])
model.graph.input.extend([new_input_tensor])
# 将模型保存为新的.onnx文件
onnx.save(model, 'new_model.onnx')
```
其中,我们首先加载了原始的.onnx模型,并获取了输入节点和输入张量的信息。然后,我们将输入节点的通道顺序从NHWC修改为NCHW,并创建了新的输入张量。接着,我们将原始的输入张量从模型中删除,并将新的输入张量添加到模型中。最后,我们将新的模型保存为新的.onnx文件。
python 使用455版本opencv写一个可以调用onnx模型的程序
首先,确保你已经安装了OpenCV 4.5.5和ONNX Runtime库。
然后,你可以使用以下代码加载ONNX模型并在OpenCV中使用它进行推理:
```python
import cv2
import numpy as np
import onnxruntime as ort
# 加载 ONNX 模型
ort_session = ort.InferenceSession("model.onnx")
# 读取图像并进行预处理
img = cv2.imread("test.jpg")
img = cv2.resize(img, (224, 224))
img = img.astype('float32') / 255.0
img = np.transpose(img, [2, 0, 1])
img = np.expand_dims(img, axis=0)
# 执行推理
inputs = {ort_session.get_inputs()[0].name: img}
outputs = ort_session.run(None, inputs)
# 处理输出结果
result = np.argmax(outputs)
# 显示结果
cv2.imshow("Result", result)
cv2.waitKey(0)
```
在这个例子中,我们假设我们的ONNX模型是一个分类器,并且输入图像大小为224x224。我们首先加载模型,然后读取图像并进行预处理。我们将图像转换为浮点数格式,并缩放到[0,1]的范围内。然后,我们将图像转换为(NCHW)格式,其中N是批次大小,C是通道数,H和W是图像高度和宽度。我们使用`np.expand_dims`函数将图像扩展为一个四维张量,批次大小为1。最后,我们将输入数据作为字典传递给ONNX运行时的`session.run`方法,并将结果转换为最终的输出结果。
注意,此代码仅用于演示目的,并且需要根据你的模型和数据进行修改。
相关推荐
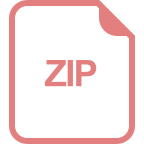
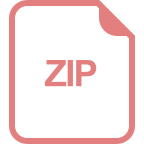
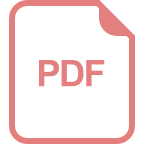













