反转一个单链表。 示例: 输入: 1->2->3->4->5->NULL 输出: 5->4->3->2->1->NULL
时间: 2023-08-07 16:55:03 浏览: 78
要反转一个单链表,你可以按照以下步骤进行:
1. 定义三个指针:prev(前一个节点)、current(当前节点)和next(下一个节点)。
2. 初始化 prev 为 nullptr,current 为链表的头节点。
3. 遍历链表,将 current 的 next 指针指向 prev,然后更新 prev、current 和 next 分别为 current、next 和 next->next。
4. 重复步骤 3,直到遍历完整个链表。
5. 最后,将链表的头节点指向 prev。
下面是一个示例的 C++ 代码实现:
```cpp
#include <iostream>
struct Node {
int data;
Node* next;
};
// 在链表末尾添加新节点
void appendNode(Node** head, int newData) {
Node* newNode = new Node();
newNode->data = newData;
newNode->next = nullptr;
if (*head == nullptr) {
*head = newNode;
return;
}
Node* temp = *head;
while (temp->next != nullptr) {
temp = temp->next;
}
temp->next = newNode;
}
// 反转链表
Node* reverseList(Node* head) {
Node* prev = nullptr;
Node* current = head;
Node* next = nullptr;
while (current != nullptr) {
next = current->next;
current->next = prev;
prev = current;
current = next;
}
return prev;
}
// 打印链表
void printList(Node* head) {
Node* temp = head;
while (temp != nullptr) {
std::cout << temp->data << " ";
temp = temp->next;
}
std::cout << std::endl;
}
int main() {
Node* head = nullptr;
// 添加一些节点
appendNode(&head, 1);
appendNode(&head, 2);
appendNode(&head, 3);
appendNode(&head, 4);
appendNode(&head, 5);
std::cout << "原始链表: ";
printList(head);
head = reverseList(head);
std::cout << "反转后的链表: ";
printList(head);
return 0;
}
```
代码中的 `reverseList` 函数使用了三个指针 prev、current 和 next 来反转链表。我们使用一个 while 循环遍历链表,每次迭代将 current 的 next 指针指向 prev,然后更新 prev、current 和 next 的值。最后,我们返回 prev,作为反转后的链表的头节点。
在 `main` 函数中,我们创建了一个示例链表,然后调用 `reverseList` 函数来反转链表,并打印结果。
希望对你有所帮助!如果有任何问题,请随时提问。
相关推荐
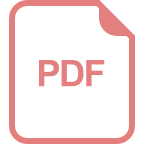
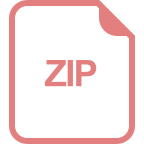
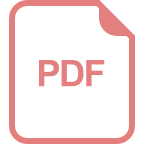














