import { ref } from 'vue'; export default { setup() { const result = ref(''); const showPicker = ref(false); const onConfirm = ({ selectedValues }) => { result.value = selectedValues.join('/'); showPicker.value = false; }; return { result, onConfirm, showPicker, }; }, };可以放在setup里面
时间: 2024-02-29 08:55:19 浏览: 104
这是一个使用 Vue 3 Composition API 的组件选项对象。在 `setup` 函数中,我们可以访问到组件的上下文对象,并且可以在其中定义响应式数据、计算属性、方法等。在这个例子中,我们使用 `ref` 函数来定义了三个响应式数据 `result`、`showPicker` 和 `onConfirm`。
`result` 用来存储选择器选中的结果,`showPicker` 控制是否显示选择器,`onConfirm` 是选择器确定按钮的回调函数,用来更新 `result` 和关闭选择器。
因此,这段代码完全可以放在 `setup` 函数中,这样它就可以被 Vue 3 正确地处理和管理了。
相关问题
<script setup lang="ts"> import { ref, toRefs, defineEmits, getCurrentInstance, watch } from "vue"; import { ElLoading, ElMessage, ElMessageBox, UploadInstance } from "element-plus"; import { auth_check, auth_token, auth_verify } from "@renderer/api/auth"; import { auth_sms, auth_scan, auth_login } from "@renderer/api"; import QrcodeVue from 'qrcode.vue' import useAuthStore from '@renderer/store/modules/auth' const emit = defineEmits(['refreshTokenVisible', 'refreshLoginErrorVisible', 'refreshQrcodeVisibleVisible']) type Props = { errorCode: string } const props = withDefaults(defineProps<Props>(), { }) const { errorCode } = toRefs(props); const tokenVisible = ref(false) const loginErrorVisible = ref(false) const qrcodeVisible = ref(false) const handleErrorCodeChange = (newV, oldV) => { console.log("newV", newV) if (newV === '1000002') { tokenVisible.value = true } else if (newV === '070004') { loginErrorVisible.value = true } else if (newV === '070001') { qrcodeVisible.value = true } } watch(() => errorCode, handleErrorCodeChange, { immediate: true } ); const authStore = useAuthStore() const validateCode = ref('' as String) const validateCodeVisible = ref(false) </script> watch监听父组件传过来的errorCode的值,没有效果
根据你提供的代码片段,`watch` 监听父组件传递过来的 `errorCode` 值应该是有效的。然而,为了确保正确地监听和处理这个值的变化,你可以尝试做以下几点调整:
1. 确保在父组件中正确传递 `errorCode` 属性给子组件。例如:
```html
<ChildComponent :errorCode="errorCode" />
```
2. 确保 `errorCode` 在父组件中是响应式的。可以使用 `ref` 或 `reactive` 来包装它。例如:
```javascript
import { ref } from 'vue';
export default {
setup() {
const errorCode = ref('');
// ...
return {
errorCode,
};
},
};
```
3. 在子组件中,将 `errorCode` 解构为 `toRefs` 对象,并使用 `watch` 监听它的变化。例如:
```javascript
import { ref, toRefs, watch } from 'vue';
export default {
props: {
errorCode: String,
},
setup(props) {
const { errorCode } = toRefs(props);
watch(errorCode, (newErrorCode, oldErrorCode) => {
console.log('errorCode 变为', newErrorCode);
// 执行相应的操作
});
// ...
return {
// ...
errorCode,
};
},
};
```
确保按照以上步骤进行设置后,`watch` 应该能够正确监听和处理 `errorCode` 的变化。如果还有问题,请提供更多相关代码以便更好地帮助你解决问题。
<script lang="ts" setup> import { ref } from 'vue' const activeIndex = ref('1') const activeIndex2 = ref('1') const handleSelect = (key: string, keyPath: string[]) => { console.log(key, keyPath) } </script>将这段代码改写为vue3的js代码,并解释其意思
下面是Vue 3的JavaScript代码:
```javascript
import { ref } from 'vue'
const activeIndex = ref('1')
const activeIndex2 = ref('1')
const handleSelect = (key, keyPath) => {
console.log(key, keyPath)
}
export default {
setup() {
return {
activeIndex,
activeIndex2,
handleSelect
}
}
}
```
这段代码首先使用了 `import` 语句导入了Vue 3的 `ref` 方法。然后,它定义了两个响应式变量 `activeIndex` 和 `activeIndex2` 并初始化为 `'1'`。接下来,它定义了一个名为 `handleSelect` 的函数,用于处理 `select` 事件并在控制台中输出选中的 `key` 和 `keyPath`。最后,使用 `setup` 函数将这些变量和函数导出,以便在组件中使用。
阅读全文
相关推荐
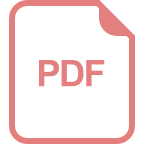
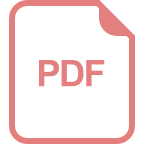
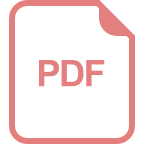













