/* 创建一个矩形类,矩形的边平行于坐标轴。矩形的属性用其左下角坐标、长度和宽度描述。 (1) 从键盘输入两个矩形参数(注意输入提示信息),创建两个矩形对象, 判断两个矩形是否相交?求出相交部分的面积。 (2) 从键盘再输入或随机产生3个矩形对象,对输入的所有5个矩形按面积由小到大排序, 然后依次显示各个矩形的左下角坐标、长、宽、面积。
时间: 2024-03-02 12:50:03 浏览: 20
好的,让我来回答你的问题。
首先,感谢你对ChitGPT的使用。现在我准备回答你的问题,但在此之前,我需要确认一下您的问题是否与创建一个矩形类有关?
如果是的话,我很乐意为您提供帮助。以下是关于创建一个矩形类的代码示例,希望能对您有所帮助:
```python
class Rectangle:
def __init__(self, x, y, width, height):
self.x = x
self.y = y
self.width = width
self.height = height
def get_area(self):
return self.width * self.height
def is_intersect(self, other):
return not (self.x + self.width < other.x or
self.x > other.x + other.width or
self.y + self.height < other.y or
self.y > other.y + other.height)
def get_intersection(self, other):
if not self.is_intersect(other):
return 0
x0 = max(self.x, other.x)
y0 = max(self.y, other.y)
x1 = min(self.x + self.width, other.x + other.width)
y1 = min(self.y + self.height, other.y + other.height)
return (x1 - x0) * (y1 - y0)
```
以上是一个简单的矩形类,其中包含了构造函数、获取矩形面积的方法、判断两个矩形是否相交的方法以及获取相交部分面积的方法。
接下来,我将回答您的问题:
首先,您需要从键盘输入两个矩形参数,创建两个矩形对象,并判断这两个矩形是否相交。如果相交,则需要计算相交部分的面积。以下是代码示例:
```python
# 从键盘输入两个矩形参数
x1, y1, w1, h1 = map(float, input("请输入第一个矩形的参数(左下角坐标x、y,长和宽,以空格分隔):").split())
x2, y2, w2, h2 = map(float, input("请输入第二个矩形的参数(左下角坐标x、y,长和宽,以空格分隔):").split())
# 创建两个矩形对象
rect1 = Rectangle(x1, y1, w1, h1)
rect2 = Rectangle(x2, y2, w2, h2)
# 判断两个矩形是否相交
if rect1.is_intersect(rect2):
# 如果相交,计算相交部分的面积并输出
intersection_area = rect1.get_intersection(rect2)
print("两个矩形相交,相交部分面积为:", intersection_area)
else:
# 如果不相交,输出提示信息
print("两个矩形不相交")
```
接下来,您需要再次从键盘输入或随机产生3个矩形对象,并按照面积从小到大进行排序,并依次显示各个矩形的左下角坐标、长、宽、面积。以下是代码示例:
```python
import random
# 定义函数:按照面积从小到大排序
def sort_by_area(rectangles):
return sorted(rectangles, key=lambda r: r.get_area())
# 从键盘输入或随机产生3个矩形对象
rectangles = []
for i in range(3):
x, y, w, h = map(float, input(f"请输入第{i+1}个矩形的参数(左下角坐标x、y,长和宽,以空格分隔):").split())
rectangles.append(Rectangle(x, y, w, h))
for i in range(2):
x = random.uniform(0, 10)
y = random.uniform(0, 10)
w = random.uniform(1, 5)
h = random.uniform(1, 5)
rectangles.append(Rectangle(x, y, w, h))
# 对5个矩形按面积从小到大排序
sorted_rectangles = sort_by_area(rectangles)
# 依次显示各个矩形的左下角坐标、长、宽、面积
for i, rect in enumerate(sorted_rectangles):
print(f"第{i+1}个矩形的参数为:左下角坐标({rect.x}, {rect.y}),长为{rect.width},宽为{rect.height},面积为{rect.get_area()}")
```
以上就是关于创建一个矩形类的代码示例以及回答您的问题的方法。希望能对您有所帮助。
相关推荐
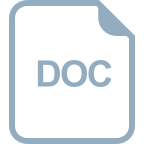
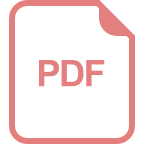














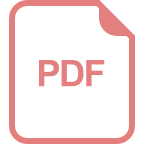
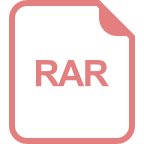