/* 创建一个矩形类,矩形的边平行于坐标轴。矩形的属性用其左下角坐标、长度和宽度描述。 (1) 从键盘输入两个矩形参数(注意输入提示信息),创建两个矩形对象, 判断两个矩形是否相交?求出相交部分的面积。 (2) 从键盘再输入或随机产生3个矩形对象,对输入的所有5个矩形按面积由小到大排序, 然后依次显示各个矩形的左下角坐标、长、宽、面积。 */
时间: 2024-03-02 15:51:32 浏览: 23
好的,我可以帮你回答这个问题。
首先,我们需要定义一个矩形类,包含左下角坐标、长度和宽度属性,以及判断是否相交和求相交部分面积的方法。
代码如下:
```python
class Rectangle:
def __init__(self, x: float, y: float, w: float, h: float):
self.x = x
self.y = y
self.w = w
self.h = h
def is_intersect(self, other: 'Rectangle') -> bool:
return not (self.x + self.w <= other.x or other.x + other.w <= self.x or
self.y + self.h <= other.y or other.y + other.h <= self.y)
def intersect_area(self, other: 'Rectangle') -> float:
if not self.is_intersect(other):
return 0.0
dx = min(self.x + self.w, other.x + other.w) - max(self.x, other.x)
dy = min(self.y + self.h, other.y + other.h) - max(self.y, other.y)
return dx * dy
```
其中 `is_intersect` 方法用于判断两个矩形是否相交,`intersect_area` 方法用于求相交部分的面积。
接下来,我们可以按照题目要求,从键盘输入两个矩形参数,创建两个矩形对象,并判断它们是否相交,如果相交则输出相交部分的面积。
代码如下:
```python
if __name__ == '__main__':
# 从键盘输入两个矩形参数
x1, y1, w1, h1 = map(float, input('请输入第一个矩形的左下角坐标、长和宽,用空格分隔:').split())
x2, y2, w2, h2 = map(float, input('请输入第二个矩形的左下角坐标、长和宽,用空格分隔:').split())
# 创建两个矩形对象
r1 = Rectangle(x1, y1, w1, h1)
r2 = Rectangle(x2, y2, w2, h2)
# 判断两个矩形是否相交,并输出相交部分的面积
if r1.is_intersect(r2):
print('两个矩形相交,相交部分的面积为:', r1.intersect_area(r2))
else:
print('两个矩形不相交')
```
接下来,我们需要从键盘或随机产生三个矩形对象,并按面积由小到大排序,然后依次显示各个矩形的左下角坐标、长、宽、面积。
代码如下:
```python
import random
if __name__ == '__main__':
# 从键盘或随机产生三个矩形对象
rectangles = []
for i in range(3):
if input('请问您是否要手动输入第 %d 个矩形的参数?(y/n) ' % (i + 1)) == 'y':
x, y, w, h = map(float, input('请输入第 %d 个矩形的左下角坐标、长和宽,用空格分隔:' % (i + 1)).split())
else:
x, y, w, h = random.uniform(0, 10), random.uniform(0, 10), random.uniform(1, 5), random.uniform(1, 5)
rectangles.append(Rectangle(x, y, w, h))
# 按面积由小到大排序
rectangles.sort(key=lambda r: r.w * r.h)
# 依次显示各个矩形的左下角坐标、长、宽、面积
for i, r in enumerate(rectangles):
print('第 %d 个矩形的左下角坐标为 (%.2f, %.2f),长为 %.2f,宽为 %.2f,面积为 %.2f' %
(i + 1, r.x, r.y, r.w, r.h, r.w * r.h))
```
这样,我们就完成了题目要求的所有功能。希望能对你有所帮助!
相关推荐
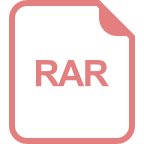
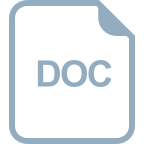
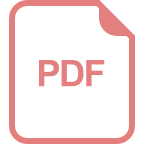














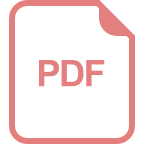
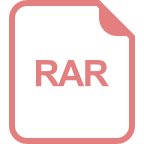