编写一段代码,内容是一个三节点的es集群
时间: 2023-09-13 13:05:19 浏览: 45
### 回答1:
以下是一段使用 Elasticsearch Java API 编写的三节点 ES 集群代码示例:
```
import org.elasticsearch.client.Client;
import org.elasticsearch.client.transport.TransportClient;
import org.elasticsearch.common.settings.Settings;
import org.elasticsearch.common.transport.TransportAddress;
import org.elasticsearch.transport.client.PreBuiltTransportClient;
import java.net.InetAddress;
public class ThreeNodeESCluster {
public static void main(String[] args) throws Exception {
// 设置集群名称
Settings settings = Settings.builder().put("cluster.name", "my-es-cluster").build();
// 创建一个 TransportClient
TransportClient client = new PreBuiltTransportClient(settings);
// 添加三个节点
client.addTransportAddress(new TransportAddress(InetAddress.getByName("node1"), 9300));
client.addTransportAddress(new TransportAddress(InetAddress.getByName("node2"), 9300));
client.addTransportAddress(new TransportAddress(InetAddress.getByName("node3"), 9300));
// 关闭客户端连接
client.close();
}
}
```
请注意,在上面的代码中,我们假设您的三个节点分别命名为 `node1`,`node2` 和 `node3`,并且每个节点的 Elasticsearch 实例在 9300 端口上监听。此外,您需要在代码中添加更多的实际操作,以完整使用 ES 集群。
### 回答2:
编写一个三节点的ES集群代码的例子如下:
```
# 导入必要的模块
from elasticsearch import Elasticsearch
# 创建一个ES集群实例
es = Elasticsearch([
{'host': '节点1的IP地址', 'port': '节点1的端口号'},
{'host': '节点2的IP地址', 'port': '节点2的端口号'},
{'host': '节点3的IP地址', 'port': '节点3的端口号'}
])
# 测试连接是否成功
if es.ping():
print("ES集群连接成功!")
else:
print("ES集群连接失败!")
# 示例:创建索引
index_name = "my_index"
index_body = {
"settings": {
"number_of_shards": 1,
"number_of_replicas": 1
},
"mappings": {
"properties": {
"title": {"type": "text"},
"content": {"type": "text"}
}
}
}
es.indices.create(index=index_name, body=index_body)
print("索引创建成功!")
```
在这个例子中,我们通过导入`elasticsearch`模块来创建一个ES集群的实例。我们需要指定每个节点的IP地址和端口号来初始化连接。然后,我们可以使用`ping()`方法来测试集群的连接是否成功。
在示例中,我们展示了如何创建一个名为`my_index`的索引。我们使用`create()`方法来指定索引名称和索引的设置和映射。在这里,我们创建了一个只有一个分片和一个副本的索引,并定义了`title`和`content`字段的数据类型。
需要注意的是,这只是一个简单的示例,实际上一个真正的ES集群还需要进行更多的配置和设置,比如使用正确的用户名和密码、配置TLS/SSL等。在实际使用中,还需要根据具体需求来编写更复杂的代码。
### 回答3:
要编写一个包含三个节点的Elasticsearch集群,可以使用以下代码:
```python
from elasticsearch import Elasticsearch
from elasticsearch.exceptions import ElasticsearchException
# 定义集群节点的主机地址和端口
nodes = [
{'host': 'node1', 'port': 9200},
{'host': 'node2', 'port': 9200},
{'host': 'node3', 'port': 9200}
]
try:
# 创建一个Elasticsearch集群对象
es = Elasticsearch(hosts=nodes)
# 检查集群的健康状态
health = es.cluster.health()
if health['status'] == 'green':
print("集群健康状态:绿色")
elif health['status'] == 'yellow':
print("集群健康状态:黄色")
elif health['status'] == 'red':
print("集群健康状态:红色")
# 查看集群中的节点信息
nodes_info = es.nodes.info()
for node_id, node_info in nodes_info['nodes'].items():
print("节点ID:", node_id)
print("主机名:", node_info['name'])
print("协议:", node_info['http_address'])
print("IP地址:", node_info['ip'])
print()
except ElasticsearchException as e:
print("连接Elasticsearch集群出错:", e)
```
这段代码使用elasticsearch-py库来与Elasticsearch集群进行通信。首先,定义了三个节点的主机地址和端口。然后,通过`Elasticsearch`类创建了一个Elasticsearch集群对象,并使用指定的节点进行连接。接下来,使用`cluster.health()`方法获取集群的健康状态,并根据状态输出相应的信息。最后,使用`nodes.info()`方法获取集群中所有节点的信息,并逐个输出每个节点的相关信息。
注意,你需要提前安装elasticsearch-py库(使用`pip install elasticsearch`命令)才能运行以上代码。
相关推荐
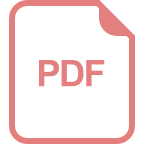
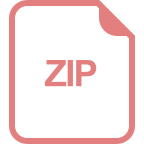
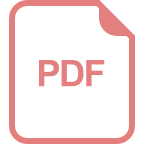
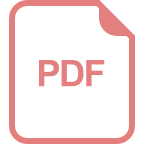
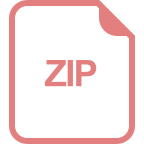
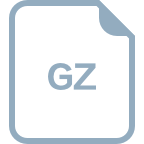
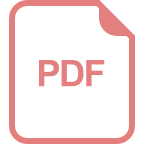
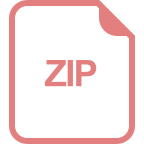
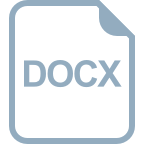
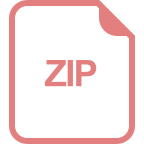
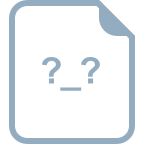
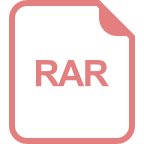