用python写一个爬虫,爬取csdn
时间: 2024-05-14 14:14:33 浏览: 12
的文章标题和链接
```python
import requests
from bs4 import BeautifulSoup
url = 'https://blog.csdn.net/'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
for article in soup.select('.article-list .title h2 a'):
print(article.text)
print(article['href'])
```
解释:
1. 首先导入`requests`和`BeautifulSoup`模块。
2. 定义要爬取的网址。
3. 发送请求并获取响应。
4. 使用`BeautifulSoup`解析响应文本。
5. 遍历文章列表中的每一篇文章,并输出标题和链接。其中,`soup.select('.article-list .title h2 a')`表示选择所有class为`article-list`和`title`的元素下的`h2`标签下的`a`标签。
相关问题
用python写一个爬取csdn首页的爬虫程序
好的,以下是一个简单的Python爬虫程序,可以爬取CSDN首页的文章标题和链接:
```python
import requests
from bs4 import BeautifulSoup
url = "https://www.csdn.net/"
response = requests.get(url)
soup = BeautifulSoup(response.content, "html.parser")
articles = soup.find_all("div", class_="title")
for article in articles:
title = article.find("a").string.strip()
link = article.find("a")["href"]
print(title)
print(link)
```
这个程序使用了`requests`库和`BeautifulSoup`库,首先通过`requests.get()`方法获取CSDN首页的HTML内容,然后使用`BeautifulSoup`解析HTML内容,找到所有标题的`div`标签,遍历每个标签,提取出标题和链接,最后输出到控制台。
写一个 python 爬取csdn首页网站代码
Sure, 这是python爬虫代码:
```python
import requests
from bs4 import BeautifulSoup
url = 'https://www.csdn.net/'
r = requests.get(url)
soup = BeautifulSoup(r.text, 'html.parser')
links = soup.find_all('a')
for link in links:
print(link.get('href'), link.text)
```
这个代码可以爬取CSDN首页的所有链接和对应的文本。希望能帮到你!
相关推荐
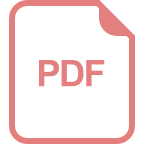
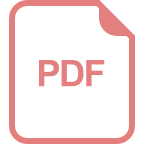












