dataframe怎么对某列数据所有行进行修改操作
时间: 2023-12-18 08:02:47 浏览: 25
可以使用DataFrame的.loc或者.iloc方法进行操作,例如:
```python
import pandas as pd
# 创建一个DataFrame
df = pd.DataFrame({
'A': [1, 2, 3],
'B': [4, 5, 6],
'C': [7, 8, 9]
})
# 使用.loc方法对某列进行修改操作
df.loc[:, 'B'] = [10, 11, 12]
# 使用.iloc方法对某列进行修改操作
df.iloc[:, 2] = [13, 14, 15]
print(df)
```
输出结果为:
```
A B C
0 1 10 13
1 2 11 14
2 3 12 15
```
相关问题
举例说明,如何对dataframe中某列中的每行的数据进行切片
要对dataframe中某列中的每行的数据进行切片,可以使用apply方法结合lambda表达式来实现。具体步骤如下:
1. 定义一个lambda函数,在函数内部使用切片操作对每个元素进行切片。
2. 使用apply方法,将上一步定义好的lambda函数应用到需要进行切片的列上。
下面是一个示例代码,假设有一个名为`df`的dataframe,其中有一个名为`text`的列,需要对每个元素进行切片,保留前5个字符:
```
df['text'] = df['text'].apply(lambda x: x[:5])
```
这段代码会将`df`中的`text`列中的每个元素都进行切片操作,只保留前5个字符。可以根据实际需求修改lambda函数内的切片操作。
如何对dataframe中的某一列进行操作
您可以使用 Pandas 库来对 dataframe 中的某一列进行操作。首先,您需要使用 Pandas 的 read_csv() 函数将数据读入 dataframe 中。然后,您可以使用 dataframe 的列名来选择要操作的列。例如,如果您要对名为 "age" 的列进行操作,您可以使用以下代码:
```python
import pandas as pd
# 读入数据
df = pd.read_csv('data.csv')
# 对 "age" 列进行操作
df['age'] = df['age'] + 1
```
在这个例子中,我们将 "age" 列中的所有值加 1。您可以根据需要使用不同的操作来修改列中的值。
相关推荐
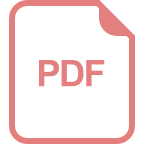
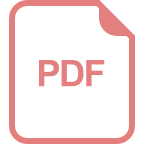
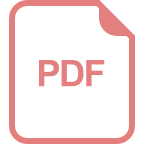












