如何自己用Python实现bayer的demosaic算法
时间: 2024-03-13 14:43:02 浏览: 168
Bayer的demosaic算法是一种基于图像传感器的色彩滤波方法。在这种方法中,相邻像素被赋予不同的颜色滤波器,如红色、绿色和蓝色滤波器。这些滤波器的排列方式决定了所使用的Bayer样式。
下面是一个简单的Python实现Bayer的demosaic算法的示例代码:
```python
import numpy as np
import cv2
def demosaic_bayer(img_bayer):
h, w = img_bayer.shape
img_rgb = np.zeros((h, w, 3), dtype=np.uint8)
# 生成掩模矩阵
mask = np.zeros((h, w), dtype=np.uint8)
mask[0::2, 0::2] = 1
mask[1::2, 1::2] = 1
# 按颜色通道分割
img_r = np.zeros((h, w), dtype=np.uint8)
img_g = np.zeros((h, w), dtype=np.uint8)
img_b = np.zeros((h, w), dtype=np.uint8)
img_r[0::2, 0::2] = img_bayer[0::2, 0::2]
img_g[0::2, 1::2] = img_bayer[0::2, 1::2]
img_g[1::2, 0::2] = img_bayer[1::2, 0::2]
img_b[1::2, 1::2] = img_bayer[1::2, 1::2]
# 对于绿色通道,使用平均值
img_g = cv2.GaussianBlur(img_g, (3, 3), 0)
# 对于红色和蓝色通道,使用双线性插值
img_r = cv2.resize(img_r, (w*2, h*2), interpolation=cv2.INTER_CUBIC)[::2, ::2]
img_b = cv2.resize(img_b, (w*2, h*2), interpolation=cv2.INTER_CUBIC)[::2, ::2]
img_rgb[..., 0] = img_r
img_rgb[..., 1] = img_g
img_rgb[..., 2] = img_b
return img_rgb
```
该函数首先生成掩模矩阵,然后将输入图像分割为红色、绿色和蓝色通道。对于绿色通道,使用高斯模糊来平滑图像。对于红色和蓝色通道,使用双线性插值来插值缺失的像素值。最后,将三个通道的图像合并成一个RGB图像并返回。
注意,这只是一个简单的实现,可能在某些情况下效果不佳。在实际应用中,可能需要更复杂的算法来获得更好的结果。
阅读全文
相关推荐
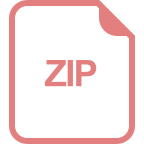
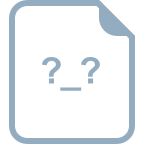















