基于OpenCV的connectedComponentsWithStats查找图像连通域,使用C++并进行从左上角到右下角的坐标排序
时间: 2024-05-12 15:19:37 浏览: 132
以下是基于OpenCV的connectedComponentsWithStats函数查找图像连通域,并按从左上角到右下角的坐标进行排序的C代码示例:
```c
#include <stdio.h>
#include <opencv2/opencv.hpp>
using namespace cv;
int main(int argc, char** argv)
{
Mat image = imread(argv[1], IMREAD_GRAYSCALE);
if (image.empty())
{
printf("Cannot read image file: %s\n", argv[1]);
return -1;
}
// Find connected components with statistics
Mat labels, stats, centroids;
int num_labels = connectedComponentsWithStats(image, labels, stats, centroids);
// Sort by top-left corner coordinates
for (int i = 1; i < num_labels; i++)
{
for (int j = i + 1; j < num_labels; j++)
{
if (stats.at<int>(i, CC_STAT_LEFT) > stats.at<int>(j, CC_STAT_LEFT) ||
(stats.at<int>(i, CC_STAT_LEFT) == stats.at<int>(j, CC_STAT_LEFT) &&
stats.at<int>(i, CC_STAT_TOP) > stats.at<int>(j, CC_STAT_TOP)))
{
// Swap labels
int temp_label = labels.at<int>(0, i);
labels.at<int>(0, i) = labels.at<int>(0, j);
labels.at<int>(0, j) = temp_label;
// Swap statistics
for (int k = 0; k < CC_STAT_MAX; k++)
{
int temp_stat = stats.at<int>(i, k);
stats.at<int>(i, k) = stats.at<int>(j, k);
stats.at<int>(j, k) = temp_stat;
}
// Swap centroids
for (int k = 0; k < 2; k++)
{
double temp_centroid = centroids.at<double>(i, k);
centroids.at<double>(i, k) = centroids.at<double>(j, k);
centroids.at<double>(j, k) = temp_centroid;
}
}
}
}
// Print statistics for each component
for (int i = 1; i < num_labels; i++)
{
printf("Component %d:\n", i);
printf(" Left: %d\n", stats.at<int>(i, CC_STAT_LEFT));
printf(" Top: %d\n", stats.at<int>(i, CC_STAT_TOP));
printf(" Width: %d\n", stats.at<int>(i, CC_STAT_WIDTH));
printf(" Height: %d\n", stats.at<int>(i, CC_STAT_HEIGHT));
printf(" Area: %d\n", stats.at<int>(i, CC_STAT_AREA));
printf(" Centroid: (%f, %f)\n", centroids.at<double>(i, 0), centroids.at<double>(i, 1));
}
return 0;
}
```
该代码使用OpenCV的connectedComponentsWithStats函数找到图像中的连通域,并使用嵌套循环按从左上角到右下角的顺序对它们进行排序。然后,对于每个连通域,该代码输出其统计信息,包括左上角坐标、宽度、高度、面积和质心。
阅读全文
相关推荐


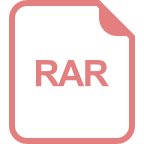
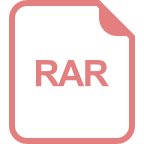
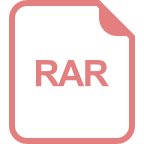
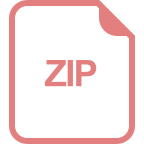
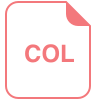








