C++怎么判断一个double类型的数字是小数点后有几位0?
时间: 2024-03-01 21:50:17 浏览: 18
可以使用C++中的字符串流(stringstream)来实现。具体步骤如下:
1. 将 double 类型的数字转化为字符串形式。
2. 使用字符串流(stringstream)将字符串形式的数字读入。
3. 判断小数点后是否有0,每次读入一个字符,如果字符是'0',则计数器加1,否则重置计数器。
4. 当遇到小数点后面的第一个非0数字时,停止计数并返回计数器的值。
以下是示例代码:
```c++
#include <iostream>
#include <sstream>
#include <string>
int countTrailingZeros(double num) {
// 将 double 类型的数字转换成字符串形式
std::stringstream ss;
ss << num;
std::string str = ss.str();
// 判断小数点后是否有0
int count = 0;
bool counting = false;
for (char c : str) {
if (counting) {
if (c == '0') {
count++;
} else {
break; // 遇到非0数字,停止计数
}
} else if (c == '.') {
counting = true;
}
}
return count;
}
int main() {
double num = 3.1400000;
int count = countTrailingZeros(num);
std::cout << "The number of trailing zeros is: " << count << std::endl;
return 0;
}
```
输出结果为:
```
The number of trailing zeros is: 5
```
相关推荐
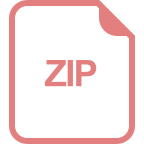













