java 修改zip中文件
时间: 2024-03-08 18:49:11 浏览: 229
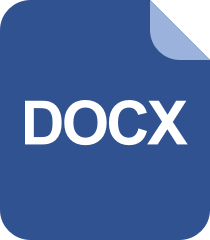
使用java代码替换zip内的文件.docx

你可以使用Java的ZipFile和ZipOutputStream类来修改一个zip文件中的文件。首先,使用ZipFile类打开zip文件,并获取到需要修改的文件的ZipEntry对象。然后,使用ZipOutputStream类创建一个新的zip文件,在其中添加修改后的文件内容。最后,将修改后的zip文件保存到磁盘上,并删除原始的zip文件。
下面是一个示例代码,演示如何使用Java修改zip文件中的文件:
```java
import java.io.*;
import java.util.zip.*;
public class ZipFileModifier {
public static void modifyFileInZip(String zipFilePath, String fileToModifyPath, String newContent) throws IOException {
// Open the zip file
File zipFile = new File(zipFilePath);
ZipFile zip = new ZipFile(zipFile);
// Get the entry for the file to modify
ZipEntry fileToModify = zip.getEntry(fileToModifyPath);
// Create a new zip file
File newZipFile = new File(zipFile.getParentFile(), "modified.zip");
ZipOutputStream out = new ZipOutputStream(new FileOutputStream(newZipFile));
// Copy all entries from the original zip file to the new zip file, except for the file to modify
Enumeration<? extends ZipEntry> entries = zip.entries();
while (entries.hasMoreElements()) {
ZipEntry entry = entries.nextElement();
if (!entry.equals(fileToModify)) {
out.putNextEntry(entry);
InputStream in = zip.getInputStream(entry);
byte[] buffer = new byte[1024];
int len;
while ((len = in.read(buffer)) > 0) {
out.write(buffer, 0, len);
}
out.closeEntry();
in.close();
}
}
// Add the modified file to the new zip file
out.putNextEntry(new ZipEntry(fileToModifyPath));
out.write(newContent.getBytes());
out.closeEntry();
// Close the streams
out.close();
zip.close();
// Delete the original zip file and rename the new zip file to the original name
if (zipFile.delete()) {
newZipFile.renameTo(zipFile);
}
}
}
```
上面的代码中,modifyFileInZip方法接受三个参数:zipFilePath表示zip文件的路径,fileToModifyPath表示需要修改的文件的路径,newContent表示修改后的文件内容。该方法会首先打开zip文件,获取到需要修改的文件的ZipEntry对象。然后,创建一个新的zip文件,并从原始zip文件中复制所有文件到新zip文件中,除了需要修改的文件。最后,将修改后的文件内容添加到新zip文件中,并将新zip文件保存到磁盘上。最后,删除原始的zip文件,并将新zip文件重命名为原始zip文件的名称。
阅读全文
相关推荐
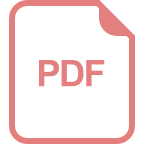
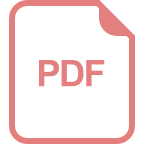















