byte数组压缩
时间: 2023-09-14 16:09:26 浏览: 42
可以使用 Java 内置的 GZIP 压缩和解压缩功能来压缩 byte 数组。下面是一个简单的示例代码:
```java
import java.io.ByteArrayInputStream;
import java.io.ByteArrayOutputStream;
import java.util.zip.GZIPInputStream;
import java.util.zip.GZIPOutputStream;
public class ByteArrayCompression {
public static byte[] compress(byte[] data) throws Exception {
ByteArrayOutputStream bos = new ByteArrayOutputStream();
GZIPOutputStream gzip = new GZIPOutputStream(bos);
gzip.write(data);
gzip.close();
byte[] compressedData = bos.toByteArray();
bos.close();
return compressedData;
}
public static byte[] decompress(byte[] compressedData) throws Exception {
ByteArrayInputStream bis = new ByteArrayInputStream(compressedData);
GZIPInputStream gis = new GZIPInputStream(bis);
ByteArrayOutputStream bos = new ByteArrayOutputStream();
byte[] buffer = new byte[1024];
int len;
while ((len = gis.read(buffer)) > 0) {
bos.write(buffer, 0, len);
}
gis.close();
bis.close();
bos.close();
return bos.toByteArray();
}
public static void main(String[] args) throws Exception {
byte[] data = "Hello, world!".getBytes();
byte[] compressedData = compress(data);
byte[] decompressedData = decompress(compressedData);
String result = new String(decompressedData);
System.out.println(result);
}
}
```
在这个例子中,我们定义了两个静态方法 `compress` 和 `decompress`,分别用于压缩和解压缩 byte 数组。我们使用 `GZIPOutputStream` 来将数据压缩为 GZIP 格式,然后使用 `GZIPInputStream` 将压缩后的数据解压缩回原始格式。最后,我们将解压缩后的数据转换为字符串并输出。
相关推荐
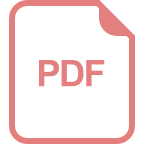
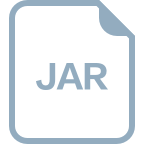














