如何使用Python读入到程序中
时间: 2024-05-07 08:22:07 浏览: 41
使用Python读取文件可以使用内置的open()函数。open()函数的第一个参数是文件名和路径,第二个参数是打开文件的模式,例如'r'表示读取模式,'w'表示写入模式。
以下是一个使用open()函数读取文件的例子:
```python
with open('file.txt', 'r') as f:
data = f.read()
print(data)
```
上面的代码将打开一个名为“file.txt”的文件,并将其内容读入变量“data”中。读取完成后,使用print()函数打印内容。
在使用open()函数时,建议使用with语句来确保文件被正确关闭。这样可以避免文件资源被占用,导致程序运行出错。
相关问题
用python编写读入Python源程序文件practice.py,删除程序中的注释后显示。
可以使用Python自带的ast模块来实现读入Python源程序文件并删除注释的功能。以下是示例代码:
```python
import ast
def remove_comments(node):
"""
删除注释节点
"""
if isinstance(node, ast.AST):
# 删除注释节点
if isinstance(node, ast.Expr) and isinstance(node.value, ast.Constant) and isinstance(node.value.value, str):
if node.value.value.startswith("#"):
return None
# 递归处理子节点
for field, old_value in ast.iter_fields(node):
if isinstance(old_value, list):
new_values = []
for value in old_value:
if isinstance(value, ast.AST):
value = remove_comments(value)
if value is not None:
new_values.append(value)
node.__setattr__(field, new_values)
elif isinstance(old_value, ast.AST):
new_value = remove_comments(old_value)
if new_value is None:
delattr(node, field)
else:
node.__setattr__(field, new_value)
return node
if __name__ == '__main__':
# 读入Python源程序文件
with open("practice.py", "r") as f:
source_code = f.read()
# 解析源程序为AST(抽象语法树)
tree = ast.parse(source_code)
# 删除注释节点
remove_comments(tree)
# 生成新的Python源程序文件
new_source_code = ast.unparse(tree)
# 显示新的Python源程序文件
print(new_source_code)
```
需要注意的是,这段代码只会删除单行注释(以`#`开头的注释),而不会删除多行注释(以`'''`或`"""`包裹的注释)。如果需要删除多行注释,可以在`remove_comments`函数中添加相应的处理逻辑。
Python读入字符串
在 Python 中,可以使用 input() 函数读取用户输入的字符串。例如:
```
name = input("请输入您的姓名:")
print("您好," + name + "!")
```
在运行上述代码时,程序会等待用户输入姓名,用户输入后按下回车键,程序会将输入的字符串赋值给变量 name,并打印出一句问候语。
除了 input() 函数外,还可以使用 open() 函数读取文件中的字符串,例如:
```
with open('file.txt', 'r') as f:
content = f.read()
print(content)
```
在运行上述代码时,程序会打开名为 file.txt 的文件,并将其中的内容读取到字符串变量 content 中,并打印出来。
阅读全文
相关推荐
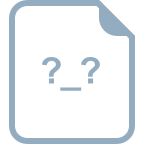
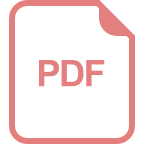








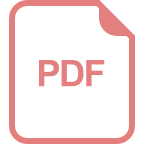




