id: r.Message.getFieldWithDefault(t, 1, "0"), shortId: r.Message.getFieldWithDefault(t, 2, "0"), nickname: r.Message.getFieldWithDefault(t, 3, ""), gender: r.Message.getFieldWithDefault(t, 4, 0), signature: r.Message.getFieldWithDefault(t, 5, ""), level: r.Message.getFieldWithDefault(t, 6, 0), birthday: r.Message.getFieldWithDefault(t, 7, "0"), telephone: r.Message.getFieldWithDefault(t, 8, ""), avatarThumb: (a = t.getAvatarThumb()) && i.Image.toObject(e, a), avatarMedium: (a = t.getAvatarMedium()) && i.Image.toObject(e, a), avatarLarge: (a = t.getAvatarLarge()) && i.Image.toObject(e, a), verified: r.Message.getBooleanFieldWithDefault(t, 12, !1), experience: r.Message.getFieldWithDefault(t, 13, 0), city: r.Message.getFieldWithDefault(t, 14, ""),这个protobuf用python写出来
时间: 2024-01-12 22:05:20 浏览: 141
在 Python 中使用 Protobuf 需要先安装 Protobuf 库,可以使用以下命令安装:
```
pip install protobuf
```
然后,需要定义protobuf的消息类型,可以使用以下代码:
```python
import protobuf
# 定义消息类型
message UserInfo {
optional string id = 1;
optional string shortId = 2;
optional string nickname = 3;
optional int32 gender = 4;
optional string signature = 5;
optional int32 level = 6;
optional string birthday = 7;
optional string telephone = 8;
optional Image avatarThumb = 9;
optional Image avatarMedium = 10;
optional Image avatarLarge = 11;
optional bool verified = 12;
optional int32 experience = 13;
optional string city = 14;
}
message Image {
optional string url = 1;
optional int32 width = 2;
optional int32 height = 3;
}
```
接下来,可以使用以下代码将 protobuf 消息类型转换为 Python 类:
```python
from protobuf_to_dict import protobuf_to_dict
# 将 protobuf 消息类型转换为 Python 类
class UserInfo(object):
def __init__(self, **kwargs):
self.id = kwargs.get('id', '')
self.shortId = kwargs.get('shortId', '')
self.nickname = kwargs.get('nickname', '')
self.gender = kwargs.get('gender', 0)
self.signature = kwargs.get('signature', '')
self.level = kwargs.get('level', 0)
self.birthday = kwargs.get('birthday', '')
self.telephone = kwargs.get('telephone', '')
self.avatarThumb = Image(**kwargs.get('avatarThumb', {}))
self.avatarMedium = Image(**kwargs.get('avatarMedium', {}))
self.avatarLarge = Image(**kwargs.get('avatarLarge', {}))
self.verified = kwargs.get('verified', False)
self.experience = kwargs.get('experience', 0)
self.city = kwargs.get('city', '')
class Image(object):
def __init__(self, **kwargs):
self.url = kwargs.get('url', '')
self.width = kwargs.get('width', 0)
self.height = kwargs.get('height', 0)
```
最后,可以使用以下代码将 protobuf 消息转换为 Python 对象:
```python
import message_pb2
# 反序列化 protobuf 消息
def deserialize_user_info(data):
user_info = message_pb2.UserInfo()
user_info.ParseFromString(data)
return protobuf_to_dict(user_info)
```
这样就可以将 protobuf 消息转换为 Python 对象了。
阅读全文
相关推荐
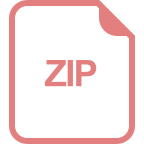
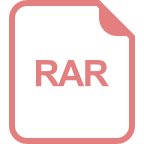
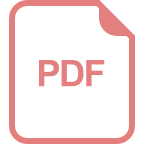
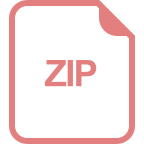















