编写一个程序,从 键盘输入一个个单词,每接收到一 个单词后,输出该单词是否曾经 出现过以及出现次次数。可以尝试分别用多重集合( multiset)和映射(map)两种途径 实现,将二者进行 比较。
时间: 2024-05-04 20:21:39 浏览: 35
使用 multiset 实现:
```cpp
#include <iostream>
#include <string>
#include <set>
int main() {
std::multiset<std::string> words;
std::string word;
while (std::cin >> word) {
if (words.find(word) == words.end()) {
std::cout << "New word: " << word << std::endl;
words.insert(word);
} else {
std::cout << "Repeated word: " << word << std::endl;
}
std::cout << "Frequency of " << word << ": " << words.count(word) << std::endl;
}
return 0;
}
```
使用 map 实现:
```cpp
#include <iostream>
#include <string>
#include <map>
int main() {
std::map<std::string, int> words;
std::string word;
while (std::cin >> word) {
if (words.find(word) == words.end()) {
std::cout << "New word: " << word << std::endl;
words[word] = 1;
} else {
std::cout << "Repeated word: " << word << std::endl;
words[word]++;
}
std::cout << "Frequency of " << word << ": " << words[word] << std::endl;
}
return 0;
}
```
在这两种实现中,我们使用了 STL 中的 multiset 和 map。multiset 是一个允许重复元素的集合,而 map 则是一种映射结构,将键值对进行关联。在 multiset 中,我们使用 count 函数来获取某个元素出现的次数;在 map 中,我们直接使用键值对来记录单词出现的次数。
相关推荐













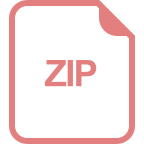