torchvision.models.resnet18修改输出类型为5
时间: 2024-04-08 15:33:00 浏览: 15
要将torchvision.models.resnet18的输出类型修改为5,你可以通过以下步骤进行修改:
1. 导入所需的库:
```python
import torch
import torchvision.models as models
```
2. 加载预训练的ResNet18模型:
```python
model = models.resnet18(pretrained=True)
```
3. 修改最后一层的全连接层,将其输出特征数修改为5:
```python
num_features = model.fc.in_features
model.fc = torch.nn.Linear(num_features, 5) # 将输出特征数修改为5
```
4. 确保模型处于训练模式(如果需要微调模型):
```python
model.train()
```
现在,你已经将ResNet18的输出类型修改为5,可以根据你的需求进行微调或训练。
相关问题
model = ResNet50(num_classes=args.num_classes).to(device) ^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^ TypeError: ResNet50.__init__() missing 1 required positional argument: 'Bottleneck'
这个错误提示表明在创建 ResNet50 模型时,缺少一个必需的位置参数“Bottleneck”。这是因为在实例化 ResNet50 模型时,需要指定使用的残差块类型。具体而言,ResNet50 中使用的是 Bottleneck 残差块。因此,需要在实例化 ResNet50 模型时传递 Bottleneck 类型。
您可以使用以下代码来实例化 ResNet50 模型:
```
import torchvision.models as models
class ResNet50(models.resnet.ResNet):
def __init__(self, num_classes):
super(ResNet50, self).__init__(
block=models.resnet.Bottleneck,
layers=[3, 4, 6, 3],
num_classes=num_classes
)
model = ResNet50(num_classes=args.num_classes).to(device)
```
在这里,我们继承了 torchvision.models.resnet.ResNet 类,并在构造函数中指定了 Bottleneck 残差块类型。同时,我们还使用了 3、4、6 和 3 个残差块的层数来构造 ResNet50 模型。最后,我们使用传递给脚本的 `num_classes` 参数来指定模型输出的类别数,并将模型移动到设备(例如 GPU)上进行加速。
举例说明 torch.export.onnx()
很抱歉,我之前给出的回答有误。实际上,PyTorch中没有名为`torch.export.onnx()`的函数。正确的函数是`torch.onnx.export()`,用于将模型导出为ONNX格式。下面是一个示例说明如何使用`torch.onnx.export()`函数:
```python
import torch
import torchvision.models as models
# 加载预训练的PyTorch模型
model = models.resnet18(pretrained=True)
# 定义输入张量(例如,输入图像)
dummy_input = torch.randn(1, 3, 224, 224)
# 导出模型为ONNX格式
torch.onnx.export(model, dummy_input, "resnet18.onnx", verbose=True)
```
在这个示例中,我们首先使用`torchvision.models`模块加载了一个预训练的ResNet-18模型。然后,我们定义了一个虚拟的输入张量`dummy_input`,它的形状是`(1, 3, 224, 224)`,表示一张RGB图像。最后,我们使用`torch.onnx.export()`函数将模型导出为ONNX格式,并指定导出的文件名为`resnet18.onnx`。通过设置`verbose=True`,我们可以获取更详细的导出过程信息。
执行上述代码后,将在当前目录下生成名为`resnet18.onnx`的ONNX模型文件,该文件可以在其他平台和框架中加载和使用。
请注意,在导出模型为ONNX格式时,可能需要对模型进行一些预处理和调整,以确保模型的兼容性和正确性。例如,您可能需要处理模型的输入和输出类型、调整模型的动态轴等。具体的处理方式取决于您的模型和使用情况。
相关推荐
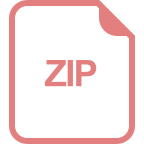
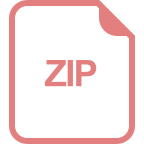












