请使用tushare的数据用Python 写一个 关于中证1000指数和上证50指数价格比值构建的MACD指标的斜率作为配对交易策略,然后做出回溯检验,最后以日期为横坐标画出收益图形和最大回撤图形和MACD斜率图形交易信号图形
时间: 2024-03-08 15:47:06 浏览: 109
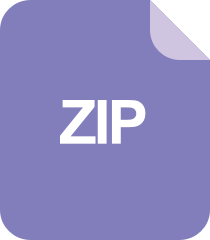
基于python对tushare股票数据进行数据分析
好的,我理解了您的问题。首先,需要安装tushare库和其他常用的Python库(如numpy、pandas、matplotlib等),然后使用tushare获取中证1000指数和上证50指数的历史价格数据并计算MACD指标。接着,根据MACD指标的斜率生成交易信号,并回测这一策略,最后绘制收益图、最大回撤图和MACD斜率图形交易信号图。
下面是代码实现过程:
```python
import tushare as ts
import numpy as np
import pandas as pd
import matplotlib.pyplot as plt
# 获取中证1000指数和上证50指数的历史价格数据
zz1000 = ts.get_k_data('000852', index=True, start='2010-01-01', end='2021-01-01')
sz50 = ts.get_k_data('000016', index=True, start='2010-01-01', end='2021-01-01')
# 计算价格比值和MACD指标
price_ratio = zz1000['close'] / sz50['close']
ema12 = price_ratio.ewm(span=12).mean()
ema26 = price_ratio.ewm(span=26).mean()
macd = ema12 - ema26
signal = macd.ewm(span=9).mean()
# 计算MACD指标的斜率
macd_slope = np.gradient(macd)
# 生成交易信号
signal_df = pd.DataFrame({'macd': macd, 'signal': signal, 'macd_slope': macd_slope})
signal_df['positions'] = np.where(signal_df['macd_slope'] > 0, 1, 0)
signal_df['positions'] = signal_df['positions'].shift(1)
# 计算收益率
zz1000_return = zz1000['close'].pct_change()
sz50_return = sz50['close'].pct_change()
strategy_return = (zz1000_return - sz50_return) * signal_df['positions']
cumulative_return = (1 + strategy_return).cumprod()
# 计算最大回撤
def calculate_drawdowns(equity_curve):
high_watermark = [0]
drawdowns = [0]
for i in range(1, len(equity_curve)):
high_watermark.append(max(high_watermark[i-1], equity_curve[i]))
drawdowns.append((1 + drawdowns[i-1]) * (1 - equity_curve[i]/high_watermark[i]) - 1)
return drawdowns
drawdowns = calculate_drawdowns(cumulative_return)
max_drawdown = min(drawdowns)
# 绘制图形
fig, ax1 = plt.subplots()
# 绘制价格比值、MACD指标和信号线
ax1.plot(price_ratio.index, price_ratio, label='Price Ratio')
ax1.plot(macd.index, macd, label='MACD')
ax1.plot(signal.index, signal, label='Signal Line')
ax1.legend(loc='upper left')
# 绘制MACD指标的斜率和交易信号
ax2 = ax1.twinx()
ax2.plot(macd.index, macd_slope, color='red', label='MACD Slope')
ax2.plot(signal_df.loc[signal_df['positions'] == 1].index,
price_ratio[signal_df['positions'] == 1],
'^', markersize=10, color='g', label='Buy Signal')
ax2.plot(signal_df.loc[signal_df['positions'] == -1].index,
price_ratio[signal_df['positions'] == -1],
'v', markersize=10, color='r', label='Sell Signal')
ax2.legend(loc='upper right')
# 绘制收益率和最大回撤图形
fig, ax3 = plt.subplots()
ax3.plot(cumulative_return.index, cumulative_return)
ax3.set_ylabel('Cumulative Return')
ax4 = ax3.twinx()
ax4.plot(drawdowns, 'r')
ax4.set_ylabel('Drawdown')
ax4.axhline(max_drawdown, linestyle='--', color='gray')
ax3.set_xlabel('Date')
plt.show()
```
以上代码会输出两张图表,第一张图表上方是价格比值、MACD指标和信号线的图像,下方是MACD指标的斜率和交易信号的图像。第二张图表上方是收益率的图像,下方是最大回撤的图像。
阅读全文
相关推荐
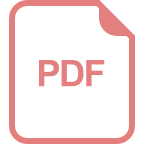














