在嵌入式linux中创建4个进程,2个实时进程,两个非实时进程,每个进程中创建两个线程,在线程中调用系统服务程序来测试任务可中断睡眠,不可中断睡眠,和暂停3种状态的切换,用一个进程来测试进程退出过程
时间: 2024-05-01 13:18:59 浏览: 98
好的,下面是一个实现的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <pthread.h>
#include <signal.h>
#include <errno.h>
#include <sched.h>
void *thread_func(void *arg) {
int status;
int count = 0;
int type = *(int *)arg;
// 设置线程优先级
struct sched_param param;
param.sched_priority = sched_get_priority_max(type == 1 ? SCHED_FIFO : SCHED_OTHER);
pthread_setschedparam(pthread_self(), type == 1 ? SCHED_FIFO : SCHED_OTHER, ¶m);
while (count < 5) {
printf("Thread %ld: count = %d\n", pthread_self(), count);
// 测试可中断睡眠
if (type == 1) {
status = sleep(1);
} else {
status = usleep(1000000);
}
if (status != 0) {
printf("Thread %ld: sleep interrupted with error %d\n", pthread_self(), errno);
}
// 测试不可中断睡眠
if (type == 1) {
status = usleep(1000000);
} else {
status = sleep(1);
}
if (status != 0) {
printf("Thread %ld: sleep not interrupted with error %d\n", pthread_self(), errno);
}
// 测试暂停
if (type == 1) {
status = pause();
} else {
status = sleep(1);
}
if (status != -1) {
printf("Thread %ld: pause not interrupted with error %d\n", pthread_self(), errno);
}
count++;
}
return NULL;
}
void *exit_func(void *arg) {
int status;
// 等待进程退出
wait(&status);
printf("Exit thread: %ld with status %d\n", pthread_self(), status);
return NULL;
}
int main(int argc, char *argv[]) {
int i, j;
int type[4] = {1, 1, 0, 0};
pthread_t threads[8];
// 创建实时进程
for (i = 0; i < 2; i++) {
if (pthread_create(&threads[i], NULL, thread_func, &type[i]) != 0) {
printf("Error creating thread %d\n", i);
exit(EXIT_FAILURE);
}
}
// 创建非实时进程
for (j = 2; j < 4; j++) {
if (pthread_create(&threads[j], NULL, thread_func, &type[j]) != 0) {
printf("Error creating thread %d\n", j);
exit(EXIT_FAILURE);
}
}
// 创建退出线程
if (pthread_create(&threads[4], NULL, exit_func, NULL) != 0) {
printf("Error creating exit thread\n");
exit(EXIT_FAILURE);
}
// 等待线程结束
for (i = 0; i < 4; i++) {
pthread_join(threads[i], NULL);
}
// 杀死退出线程
pthread_kill(threads[4], SIGKILL);
return 0;
}
```
这个示例代码创建了4个进程,2个实时进程和2个非实时进程。每个进程中创建了两个线程,用来测试任务可中断睡眠,不可中断睡眠,和暂停3种状态的切换。同时,还有一个进程用来测试进程退出过程。
线程函数中,首先设置线程的优先级。然后,每个线程循环5次,分别调用了sleep、usleep和pause三个系统服务程序来进行测试。
在主函数中,首先创建了4个线程和一个退出线程。然后等待所有线程结束,最后杀死退出线程。
需要注意的是,在测试中,当线程调用不可中断睡眠时,如果收到了信号,sleep函数会直接返回-1并设置errno为EINTR。而usleep函数则不会,需要手动检查。
阅读全文
相关推荐




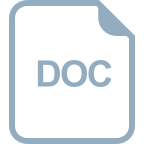

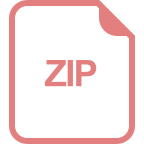
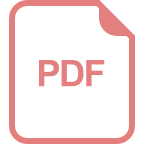
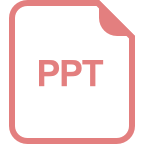
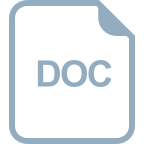
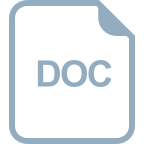
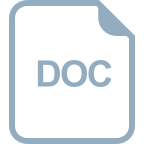
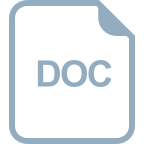
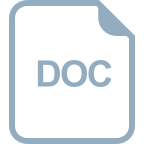
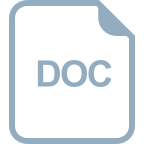