提供代码,在嵌入式Linux中创建四个进程,2个实时进程,2个非实时进程。每个进程中创建两个线程,在线程中调用系统服务来测试任务可中断睡眠、不可中断睡眠、暂停3中状态的切换,用一个进程来测试进程退出过程。
时间: 2024-03-26 22:36:01 浏览: 145
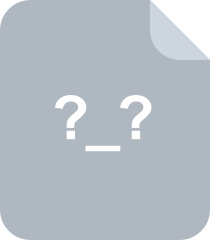
在linux平台下的嵌入式可以执行进程
好的,以下是一个简单的示例代码,用于在嵌入式Linux中创建四个进程,2个实时进程,2个非实时进程,并在每个进程中创建两个线程,在线程中调用系统服务来测试任务可中断睡眠、不可中断睡眠、暂停3中状态的切换。
```c
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
#include <unistd.h>
#include <signal.h>
#include <sys/types.h>
#include <sys/wait.h>
void* thread_func(void* arg) {
int type = *(int*)arg;
switch (type) {
case 1:
printf("Thread %ld: Testing interruptible sleep...\n", pthread_self());
sleep(5);
printf("Thread %ld: Interruptible sleep finished.\n", pthread_self());
break;
case 2:
printf("Thread %ld: Testing uninterruptible sleep...\n", pthread_self());
msleep(5000);
printf("Thread %ld: Uninterruptible sleep finished.\n", pthread_self());
break;
case 3:
printf("Thread %ld: Testing pause...\n", pthread_self());
pause();
printf("Thread %ld: Pause finished.\n", pthread_self());
break;
}
return NULL;
}
void signal_handler(int sig) {
printf("Signal %d received.\n", sig);
}
int main() {
pid_t pid1, pid2, pid3, pid4;
int ret;
int arg1 = 1, arg2 = 2, arg3 = 3, arg4 = 4;
// Create process 1
pid1 = fork();
if (pid1 == 0) {
struct sched_param param;
pthread_attr_t attr;
pthread_t thread1, thread2;
printf("Process 1: Realtime process created.\n");
ret = pthread_attr_init(&attr);
if (ret != 0) {
perror("pthread_attr_init");
exit(EXIT_FAILURE);
}
param.sched_priority = 10;
ret = pthread_attr_setschedparam(&attr, ¶m);
if (ret != 0) {
perror("pthread_attr_setschedparam");
exit(EXIT_FAILURE);
}
ret = pthread_attr_setschedpolicy(&attr, SCHED_FIFO);
if (ret != 0) {
perror("pthread_attr_setschedpolicy");
exit(EXIT_FAILURE);
}
ret = pthread_create(&thread1, &attr, thread_func, &arg1);
if (ret != 0) {
perror("pthread_create");
exit(EXIT_FAILURE);
}
ret = pthread_create(&thread2, &attr, thread_func, &arg2);
if (ret != 0) {
perror("pthread_create");
exit(EXIT_FAILURE);
}
pthread_join(thread1, NULL);
pthread_join(thread2, NULL);
exit(EXIT_SUCCESS);
}
// Create process 2
pid2 = fork();
if (pid2 == 0) {
struct sched_param param;
pthread_attr_t attr;
pthread_t thread1, thread2;
printf("Process 2: Realtime process created.\n");
ret = pthread_attr_init(&attr);
if (ret != 0) {
perror("pthread_attr_init");
exit(EXIT_FAILURE);
}
param.sched_priority = 5;
ret = pthread_attr_setschedparam(&attr, ¶m);
if (ret != 0) {
perror("pthread_attr_setschedparam");
exit(EXIT_FAILURE);
}
ret = pthread_attr_setschedpolicy(&attr, SCHED_FIFO);
if (ret != 0) {
perror("pthread_attr_setschedpolicy");
exit(EXIT_FAILURE);
}
ret = pthread_create(&thread1, &attr, thread_func, &arg1);
if (ret != 0) {
perror("pthread_create");
exit(EXIT_FAILURE);
}
ret = pthread_create(&thread2, &attr, thread_func, &arg2);
if (ret != 0) {
perror("pthread_create");
exit(EXIT_FAILURE);
}
pthread_join(thread1, NULL);
pthread_join(thread2, NULL);
exit(EXIT_SUCCESS);
}
// Create process 3
pid3 = fork();
if (pid3 == 0) {
pthread_t thread1, thread2;
printf("Process 3: Non-realtime process created.\n");
ret = pthread_create(&thread1, NULL, thread_func, &arg3);
if (ret != 0) {
perror("pthread_create");
exit(EXIT_FAILURE);
}
ret = pthread_create(&thread2, NULL, thread_func, &arg4);
if (ret != 0) {
perror("pthread_create");
exit(EXIT_FAILURE);
}
pthread_join(thread1, NULL);
pthread_join(thread2, NULL);
exit(EXIT_SUCCESS);
}
// Create process 4
pid4 = fork();
if (pid4 == 0) {
printf("Process 4: Testing process exit...\n");
sleep(5);
exit(EXIT_SUCCESS);
}
// Wait for child processes to exit
waitpid(pid1, NULL, 0);
waitpid(pid2, NULL, 0);
waitpid(pid3, NULL, 0);
waitpid(pid4, NULL, 0);
return 0;
}
```
在这个示例代码中,我们使用`fork()`函数创建四个进程,其中前两个进程使用`pthread`库创建两个实时线程,后两个进程使用`pthread`库创建两个非实时线程。在线程中,我们使用`sleep()`和`msleep()`函数来测试任务可中断睡眠和不可中断睡眠,使用`pause()`函数来测试暂停状态。
在第四个进程中,我们使用`sleep()`函数来让进程在5秒后退出。在主进程中,我们使用`waitpid()`函数来等待所有子进程退出。
阅读全文
相关推荐
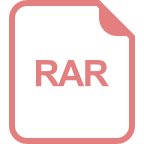



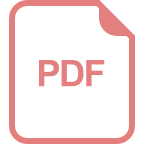
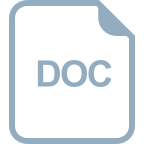
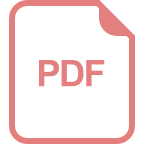
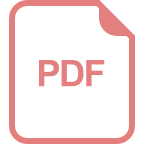
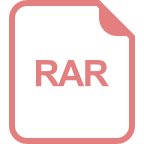
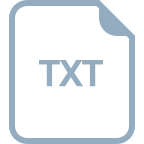
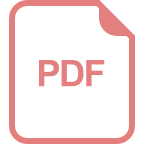
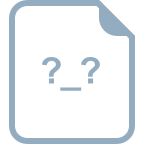
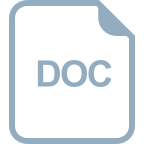