实现图的存储,输出存储结构示意图; 输出对建立的图进行深度优先搜索和广度优先搜索所得的遍历序列。(c语言邻接表)
时间: 2024-02-13 14:04:36 浏览: 83
好的,邻接表是一种常见的图的存储结构。它将每个顶点的所有邻接点存储在一个链表中,因此可以很容易地遍历每个顶点的邻接点。下面是一个邻接表的存储结构示意图:
```
typedef struct node {
int vertex; // 邻接点的顶点编号
struct node* next; // 指向下一个邻接点的指针
} Node;
typedef struct graph {
int num_vertices; // 图中顶点的数量
Node** adj_lists; // 存储所有邻接链表的指针数组
bool* visited; // 记录每个顶点是否被访问过
} Graph;
```
在这个结构中,`num_vertices` 表示图中顶点的数量,`adj_lists` 是一个指针数组,每个元素指向一个链表,存储某个顶点的所有邻接点。`visited` 数组用于记录每个顶点是否被访问过。
下面是一个简单的邻接表实现深度优先搜索和广度优先搜索的示例代码:
```
// 深度优先搜索
void dfs(Graph* graph, int vertex) {
printf("%d ", vertex);
graph->visited[vertex] = true;
Node* adj_list = graph->adj_lists[vertex];
while (adj_list != NULL) {
int adj_vertex = adj_list->vertex;
if (!graph->visited[adj_vertex]) {
dfs(graph, adj_vertex);
}
adj_list = adj_list->next;
}
}
// 广度优先搜索
void bfs(Graph* graph, int start_vertex) {
Queue* queue = create_queue();
enqueue(queue, start_vertex);
graph->visited[start_vertex] = true;
while (!is_empty(queue)) {
int vertex = dequeue(queue);
printf("%d ", vertex);
Node* adj_list = graph->adj_lists[vertex];
while (adj_list != NULL) {
int adj_vertex = adj_list->vertex;
if (!graph->visited[adj_vertex]) {
graph->visited[adj_vertex] = true;
enqueue(queue, adj_vertex);
}
adj_list = adj_list->next;
}
}
}
```
在这个示例代码中,`dfs` 函数和 `bfs` 函数分别实现了深度优先搜索和广度优先搜索。它们都接受一个 `Graph` 结构体指针和一个起始顶点作为参数。在遍历过程中,我们使用 `visited` 数组记录每个顶点是否被访问过。对于每个顶点,我们先输出它的编号,然后遍历它的邻接点,并递归地进行深度优先搜索或将邻接点加入队列进行广度优先搜索。
阅读全文
相关推荐
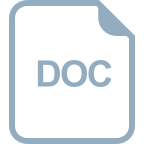
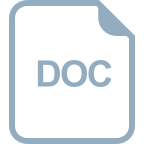
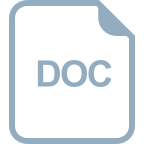















