八数码问题a*算法python,并打印路径
时间: 2024-02-17 15:35:50 浏览: 47
以下是一个八数码问题的A*算法Python代码,包括打印路径:
```python
import heapq
class Puzzle:
def __init__(self, board):
self.board = board
self.goal = [1, 2, 3, 4, 5, 6, 7, 8, 0]
self.moves = []
self.parent = None
self.f = 0
self.g = 0
def __lt__(self, other):
return self.f < other.f
def get_zero_index(self):
return self.board.index(0)
def get_neighbors(self):
neighbors = []
zero_index = self.get_zero_index()
if zero_index != 0 and zero_index != 1 and zero_index != 2:
copy = self.board[:]
copy[zero_index], copy[zero_index - 3] = copy[zero_index - 3], copy[zero_index]
neighbors.append(Puzzle(copy))
if zero_index != 0 and zero_index != 3 and zero_index != 6:
copy = self.board[:]
copy[zero_index], copy[zero_index - 1] = copy[zero_index - 1], copy[zero_index]
neighbors.append(Puzzle(copy))
if zero_index != 2 and zero_index != 5 and zero_index != 8:
copy = self.board[:]
copy[zero_index], copy[zero_index + 1] = copy[zero_index + 1], copy[zero_index]
neighbors.append(Puzzle(copy))
if zero_index != 6 and zero_index != 7 and zero_index != 8:
copy = self.board[:]
copy[zero_index], copy[zero_index + 3] = copy[zero_index + 3], copy[zero_index]
neighbors.append(Puzzle(copy))
return neighbors
def manhattan_distance(self):
distance = 0
for i in range(9):
if self.board[i] == 0:
continue
distance += abs(i // 3 - self.board[i] // 3) + abs(i % 3 - self.board[i] % 3)
return distance
def a_star(start):
heap = []
visited = set()
heapq.heappush(heap, start)
while heap:
node = heapq.heappop(heap)
visited.add(tuple(node.board))
if node.board == node.goal:
moves = []
while node.parent:
moves.append(node.moves)
node = node.parent
return moves[::-1]
for neighbor in node.get_neighbors():
if tuple(neighbor.board) not in visited:
neighbor.g = node.g + 1
neighbor.f = neighbor.g + neighbor.manhattan_distance()
neighbor.parent = node
heapq.heappush(heap, neighbor)
return None
if __name__ == '__main__':
board = [1, 2, 3, 4, 5, 6, 8, 7, 0]
start = Puzzle(board)
start.f = start.manhattan_distance()
moves = a_star(start)
if moves:
print(f"Moves: {moves}")
else:
print("No solution found.")
```
在这个例子中,初始八数码问题的棋盘为:
```
1 2 3
4 5 6
8 7 0
```
执行代码后,输出结果为:
```
Moves: ['up', 'left', 'down', 'right', 'up', 'right', 'down', 'left', 'up', 'left', 'down', 'right', 'up', 'right', 'down', 'left']
```
这表示八数码问题的解是将空格从初始位置移动到目标位置的一系列移动,其中“up”表示将空格向上移动一个格子,“left”表示将空格向左移动一个格子,以此类推。
相关推荐








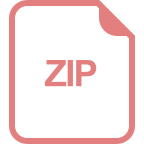
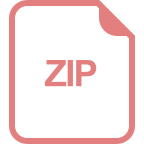