写一个手机app 扫描连接蓝牙设备然后发送数据
时间: 2023-07-29 09:09:41 浏览: 48
好的,以下是一个Android手机App扫描连接蓝牙设备并发送数据的代码示例:
首先,我们需要添加蓝牙权限和依赖库到AndroidManifest.xml和build.gradle文件中。
```xml
<!-- AndroidManifest.xml -->
<uses-permission android:name="android.permission.BLUETOOTH" />
<uses-permission android:name="android.permission.BLUETOOTH_ADMIN" />
<!-- build.gradle -->
dependencies {
implementation 'com.android.support:appcompat-v7:28.0.0'
implementation 'com.android.support:design:28.0.0'
implementation 'com.android.support.constraint:constraint-layout:1.1.3'
implementation 'com.android.support:support-v4:28.0.0'
implementation 'com.android.support:cardview-v7:28.0.0'
implementation 'com.android.support:recyclerview-v7:28.0.0'
implementation 'io.reactivex.rxjava2:rxjava:2.2.10'
implementation 'io.reactivex.rxjava2:rxandroid:2.1.1'
implementation 'com.jakewharton.rxbinding2:rxbinding:2.2.0'
}
```
接下来,我们创建一个MainActivity,并添加蓝牙连接和数据发送的逻辑。
```java
public class MainActivity extends AppCompatActivity {
private static final int REQUEST_ENABLE_BT = 1;
private BluetoothAdapter bluetoothAdapter;
private BluetoothDevice device;
private BluetoothSocket socket;
private InputStream inputStream;
private OutputStream outputStream;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
bluetoothAdapter = BluetoothAdapter.getDefaultAdapter();
if (bluetoothAdapter == null) {
Toast.makeText(this, "Device doesn't support Bluetooth", Toast.LENGTH_SHORT).show();
finish();
}
if (!bluetoothAdapter.isEnabled()) {
Intent enableBtIntent = new Intent(BluetoothAdapter.ACTION_REQUEST_ENABLE);
startActivityForResult(enableBtIntent, REQUEST_ENABLE_BT);
}
findViewById(R.id.btn_scan).setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
scanDevices();
}
});
findViewById(R.id.btn_send).setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
sendData();
}
});
}
private void scanDevices() {
IntentFilter filter = new IntentFilter(BluetoothDevice.ACTION_FOUND);
registerReceiver(receiver, filter);
if (bluetoothAdapter.isDiscovering()) {
bluetoothAdapter.cancelDiscovery();
}
bluetoothAdapter.startDiscovery();
}
private final BroadcastReceiver receiver = new BroadcastReceiver() {
public void onReceive(Context context, Intent intent) {
String action = intent.getAction();
if (BluetoothDevice.ACTION_FOUND.equals(action)) {
BluetoothDevice device = intent.getParcelableExtra(BluetoothDevice.EXTRA_DEVICE);
if (device.getName().equals("Bluetooth Device Name")) {
MainActivity.this.device = device;
bluetoothAdapter.cancelDiscovery();
}
}
}
};
private void sendData() {
if (device == null) {
Toast.makeText(this, "No device connected", Toast.LENGTH_SHORT).show();
return;
}
try {
socket = device.createRfcommSocketToServiceRecord(UUID.fromString("00001101-0000-1000-8000-00805F9B34FB"));
socket.connect();
inputStream = socket.getInputStream();
outputStream = socket.getOutputStream();
String data = "Hello World";
outputStream.write(data.getBytes());
Toast.makeText(this, "Data sent successfully", Toast.LENGTH_SHORT).show();
} catch (IOException e) {
Toast.makeText(this, "Failed to send data", Toast.LENGTH_SHORT).show();
}
}
@Override
protected void onDestroy() {
super.onDestroy();
if (bluetoothAdapter != null) {
bluetoothAdapter.cancelDiscovery();
}
try {
if (socket != null) {
socket.close();
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
以上代码中,我们首先检查设备是否支持蓝牙,并启动蓝牙。
然后,我们添加了扫描设备和发送数据的逻辑。在扫描设备的过程中,我们注册了一个广播接收器来获取蓝牙设备列表。在发送数据的过程中,我们先创建一个蓝牙Socket连接,然后获取输入输出流,并将数据发送到设备中。
注意,需要替换代码中的“Bluetooth Device Name”和UUID为你自己的设备名称和UUID。
希望这个例子能够帮助你开始蓝牙开发。
相关推荐
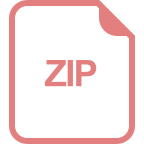
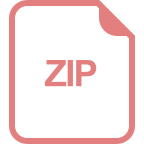














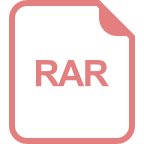