for(map<int,int>::iterator it=m.begin(); it<m.end(); it++)这句代码哪里有问题
时间: 2024-04-08 12:29:11 浏览: 15
这句代码没有明显的语法错误,但是在循环条件中使用`<`运算符可能会导致错误。`map`容器的迭代器不支持直接的小于操作符比较,因为`map`是按照键的顺序进行排序的。
正确的做法是使用`!=`运算符来比较迭代器是否到达`end()`位置,即修改为:
```cpp
for(map<int, int>::iterator it = m.begin(); it != m.end(); it++)
```
或者可以使用范围基于的循环来遍历`map`容器:
```cpp
for(auto& pair : m)
{
// 使用pair.first和pair.second访问键值对
}
```
这样可以更简洁地遍历`map`容器的元素。
相关问题
map<int, string>::iterator iter;
这是一个迭代器,用于遍历 `map<int, string>` 这个键值对容器中的元素。
具体来说,`map<int, string>` 是一个关联式容器,存储了一组键值对,其中键为 int 类型,值为 string 类型。而 `map<int, string>::iterator` 是一个迭代器类型,用于遍历这个容器中的元素。可以通过迭代器来访问容器中的元素,包括键和值。
在使用时,可以通过 `map<int, string>::iterator` 声明一个迭代器对象,例如:
```c++
map<int, string> my_map;
my_map[1] = "hello";
my_map[2] = "world";
// 使用迭代器遍历容器中的元素
map<int, string>::iterator iter;
for (iter = my_map.begin(); iter != my_map.end(); iter++) {
cout << iter->first << ": " << iter->second << endl;
}
```
在上面的示例代码中,使用迭代器 `iter` 遍历了 `my_map` 容器中的元素,并输出了每个元素的键和值。其中,`iter->first` 表示当前迭代器所指向的元素的键,`iter->second` 表示当前元素的值。
std::map<float, int>::iterator it = hist.begin(); it != hist.end() - 1; 为什么第二句话报错
### 回答1:
std::map<float, int>::iterator 是一个迭代器, 它指向 std::map<float, int> 类型的容器中的元素.
it = hist.begin() 表示将迭代器 it 指向容器 hist 的第一个元素.
it != hist.end() - 1 中, hist.end() 表示容器 hist 的最后一个元素的下一位置, 即 hist 的末尾迭代器, -1 是不合法的,所以会报错.
迭代器的使用有一些限制,在某些容器中,如std::map,std::set,其end()返回的迭代器是指向最后一个元素下一个位置的迭代器,不能使用运算符-1.
### 回答2:
第二句话报错是因为当我们尝试对 `std::map<float, int>::iterator` 类型的迭代器对象进行减法操作时,是不合法的操作。
`hist.end()` 返回的是指向 `std::map` 容器中最后一个元素之后的迭代器,它是一个 `std::map<float, int>::iterator` 类型的对象。
减去整数1并不是对迭代器进行下标运算,而是试图将一个整数值减去一个迭代器类型的对象,这是非法的操作。
如果想要到达 `hist.end()` 前一个元素的位置,可以使用 `std::prev` 函数,类似这样:`--std::prev(hist.end());`。
所以,正确的写法应该是:`std::map<float, int>::iterator it = hist.begin(); it != std::prev(hist.end());`
### 回答3:
第二句话报错是因为`hist.end()`返回的是`map`中最后一个元素的下一个位置的迭代器,而不是最后一个元素的迭代器。因此,对迭代器进行减法操作是非法的,会导致编译错误。
若想遍历整个`std::map`,应该使用`it != hist.end()`,而不是`it != hist.end() - 1`。这样可以确保遍历到最后一个元素。
示例代码如下:
```cpp
std::map<float, int>::iterator it = hist.begin();
for (; it != hist.end(); ++it) {
// 遍历代码
}
```
以上代码将遍历整个`hist`中的所有元素。
相关推荐
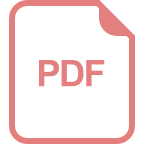
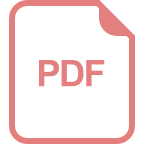













