map<string, int>::iterator
时间: 2023-12-27 21:46:25 浏览: 30
An iterator is a pointer-like object that points to an element in a container. In this case, the container is a map of strings to integers.
The iterator is of type `map<string, int>::iterator`, which means it is specifically designed to iterate through the elements of a map with string keys and integer values.
Using this iterator, you can traverse the map, access its elements, and modify them if necessary. For example, you can use the iterator to add a new element to the map, remove an existing element, or update the value of an element.
Here's an example of how to use a `map<string, int>::iterator` to iterate through a map and print out its contents:
```
#include <iostream>
#include <map>
#include <string>
using namespace std;
int main() {
map<string, int> myMap = {{"apple", 3}, {"banana", 2}, {"orange", 5}};
// Iterate through the map using an iterator
for (map<string, int>::iterator it = myMap.begin(); it != myMap.end(); ++it) {
cout << it->first << ": " << it->second << endl;
}
return 0;
}
```
In this example, the `myMap.begin()` function returns an iterator pointing to the first element of the map, and the `myMap.end()` function returns an iterator pointing to the "past-the-end" element of the map.
The `it->first` and `it->second` notation is used to access the key and value of the current element pointed to by the iterator.
The output of this program would be:
```
apple: 3
banana: 2
orange: 5
```
相关推荐
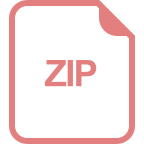
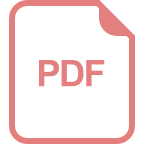
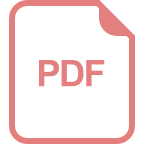















