NAO机器人采用opencv视觉识别进行自主巡黑线,可以直走与拐弯以及识别到黑色横线停止运动的python2代码
时间: 2024-02-11 10:08:44 浏览: 149
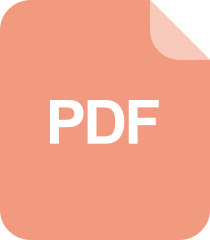
python程序控制NAO机器人行走

以下是一个简单的Python2代码示例,使用OpenCV库进行黑线跟踪和控制NAO机器人的运动:
```python
import cv2
import numpy as np
from naoqi import ALProxy
# Connect to NAO robot
IP = "NAO_IP"
PORT = 9559
motionProxy = ALProxy("ALMotion", IP, PORT)
postureProxy = ALProxy("ALRobotPosture", IP, PORT)
videoProxy = ALProxy("ALVideoDevice", IP, PORT)
# Set up camera
camera = 0
resolution = 2 # VGA
colorSpace = 11 # RGB
fps = 30
nameId = videoProxy.subscribe("python_GVM", resolution, colorSpace, fps)
print "Subscribed to camera"
# Set up black line tracking
lower_black = np.array([0,0,0])
upper_black = np.array([100,100,100])
kernel = np.ones((5,5),np.uint8)
# Start walking
motionProxy.wakeUp()
postureProxy.goToPosture("StandInit", 0.5)
while True:
# Get image from camera
image = videoProxy.getImageRemote(nameId)
if image is None:
continue
image = np.reshape(image[6], (480, 640, 3))
# Convert to grayscale and threshold
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
_, thresh = cv2.threshold(gray, 0, 255, cv2.THRESH_BINARY+cv2.THRESH_OTSU)
# Apply morphology operations
thresh = cv2.morphologyEx(thresh, cv2.MORPH_OPEN, kernel)
thresh = cv2.morphologyEx(thresh, cv2.MORPH_CLOSE, kernel)
# Find contours of black line
contours, _ = cv2.findContours(thresh, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)[-2:]
if len(contours) > 0:
# Get bounding box of largest contour
c = max(contours, key=cv2.contourArea)
x, y, w, h = cv2.boundingRect(c)
# Draw bounding box
cv2.rectangle(image, (x,y), (x+w,y+h), (0,255,0), 2)
# Continue moving forward if line is straight
if w > 200:
motionProxy.move(0.1, 0, 0)
# Turn left if line turns left
elif x < 200:
motionProxy.move(0.1, 0, 0.5)
# Turn right if line turns right
elif x > 440:
motionProxy.move(0.1, 0, -0.5)
else:
# Stop if line is lost
motionProxy.stop()
# Display image
cv2.imshow("NAO Camera", image)
key = cv2.waitKey(1) & 0xFF
if key == ord("q"):
break
# Clean up
videoProxy.unsubscribe(nameId)
cv2.destroyAllWindows()
```
该示例代码将订阅NAO机器人的摄像头,并使用OpenCV库进行图像处理。机器人将跟踪黑色线条并根据线条的位置和方向控制其运动。当机器人识别不到线条时,它将停止运动。请注意,此代码可能需要根据您的具体情况进行修改,例如IP地址和端口号。
阅读全文
相关推荐















