NAO机器人基于python的opencv走黑线的代码
时间: 2023-10-11 20:05:30 浏览: 218
以下是基于Python和OpenCV的NAO机器人走黑线的代码:
```python
import numpy as np
import cv2
import time
from naoqi import ALProxy
# NAOqi robot IP address and port
NAO_IP = "192.168.1.101"
NAO_PORT = 9559
# Initialize NAOqi proxies
motionProxy = ALProxy("ALMotion", NAO_IP, NAO_PORT)
postureProxy = ALProxy("ALRobotPosture", NAO_IP, NAO_PORT)
cameraProxy = ALProxy("ALVideoDevice", NAO_IP, NAO_PORT)
# Set camera parameters
resolution = 2 # VGA resolution
colorSpace = 11 # RGB color space
fov = 47.64 # Field of view
cameraID = 0 # Camera ID
fps = 30 # Frames per second
nameId = cameraProxy.subscribeCamera("PythonClient", cameraID, resolution, colorSpace, fps)
# Set up constants
ROBOT_VELOCITY = 0.2
BLACK_THRESHOLD = 50
# Set up initial robot position
motionProxy.wakeUp()
postureProxy.goToPosture("StandInit", 0.5)
# Main loop
while True:
# Get image from camera
imgData = cameraProxy.getImageRemote(nameId)
width = imgData[0]
height = imgData[1]
image = np.zeros((height, width, 3), np.uint8)
image.data = imgData[6]
image = cv2.cvtColor(image, cv2.COLOR_RGB2GRAY)
# Threshold image to get binary black/white image
_, thresh = cv2.threshold(image, BLACK_THRESHOLD, 255, cv2.THRESH_BINARY)
# Find contours in binary image
contours, _ = cv2.findContours(thresh, cv2.RETR_TREE, cv2.CHAIN_APPROX_SIMPLE)
# If no contours are found, stop robot
if len(contours) == 0:
motionProxy.stopMove()
else:
# Find centroid of largest contour
largestContour = max(contours, key=cv2.contourArea)
M = cv2.moments(largestContour)
cx = int(M['m10']/M['m00'])
cy = int(M['m01']/M['m00'])
# If centroid is on the left, turn left
if cx < width/2:
motionProxy.move(-ROBOT_VELOCITY, 0, 0)
# If centroid is on the right, turn right
else:
motionProxy.move(ROBOT_VELOCITY, 0, 0)
# Display image
cv2.imshow("Image", image)
cv2.waitKey(1)
# Clean up
cameraProxy.unsubscribe(nameId)
cv2.destroyAllWindows()
motionProxy.rest()
```
该代码使用了NAOqi SDK中的ALProxy类来连接到NAO机器人的运动、姿势和相机模块。然后,它从相机中获取图像,并将其转换为灰度图像。接下来,它将二进制化的黑白图像阈值化,以便能够检测到黑线的存在。然后,它找到最大的轮廓,并计算其质心。如果质心在图像的左侧,则机器人向左转,否则机器人向右转。最后,它显示了原始图像,并在循环结束时进行了清理。
阅读全文
相关推荐















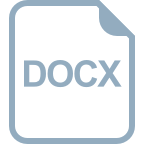