c语言利用友元函数,求任意一点到任意一条直线的距离。 编写一个程序,已有若干学生的数据,包括学号、姓名、成绩,要求输出这些学生的数据并计算出学生人数和平均成绩(要求将学生人数和总成绩用静态数据成员表示)
时间: 2024-01-05 12:02:01 浏览: 120
首先,关于友元函数求点到直线的距离,可以考虑以下代码:
```
#include <iostream>
#include <cmath>
using namespace std;
class Point {
private:
double x, y;
public:
Point(double _x, double _y) : x(_x), y(_y) {}
friend double dist(Point p, Line l);
};
class Line {
private:
double a, b, c;
public:
Line(double _a, double _b, double _c) : a(_a), b(_b), c(_c) {}
friend double dist(Point p, Line l);
};
double dist(Point p, Line l) {
return abs(l.a * p.x + l.b * p.y + l.c) / sqrt(l.a * l.a + l.b * l.b);
}
int main() {
Point p(1, 2);
Line l(3, 4, 5);
cout << dist(p, l) << endl;
return 0;
}
```
以上代码定义了一个点类和一条直线类,点类有 x、y 坐标,直线类有一般式的系数 a、b、c,然后定义了一个友元函数 dist,用于计算点到直线的距离。
接下来,关于学生数据的输出和平均成绩的计算,可以考虑以下代码:
```
#include <iostream>
#include <string>
using namespace std;
class Student {
private:
static int count;
static double totalScore;
int id;
string name;
double score;
public:
Student(int _id, string _name, double _score) : id(_id), name(_name), score(_score) {
count++;
totalScore += _score;
}
static void printStatic() {
cout << "Student count: " << count << endl;
cout << "Total score: " << totalScore << endl;
cout << "Average score: " << totalScore / count << endl;
}
void print() {
cout << "ID: " << id << ", Name: " << name << ", Score: " << score << endl;
}
};
int Student::count = 0;
double Student::totalScore = 0;
int main() {
Student s1(1, "Alice", 90);
Student s2(2, "Bob", 80);
Student s3(3, "Charlie", 70);
s1.print();
s2.print();
s3.print();
Student::printStatic();
return 0;
}
```
以上代码定义了一个学生类,其中包括学号、姓名、成绩等数据成员,以及一个静态的学生数和成绩总和数据成员。构造函数中每次添加一个学生时,都会更新静态数据成员。然后定义了一个静态函数 printStatic,用于输出学生数、总成绩和平均成绩。最后在 main 函数中创建了若干个学生对象,并输出它们的数据和计算平均成绩。
阅读全文
相关推荐
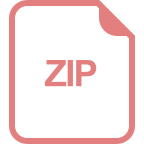
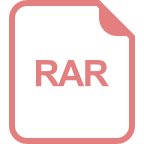
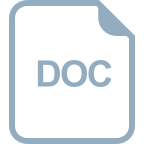
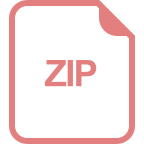
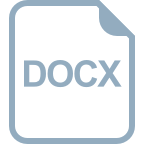
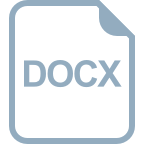
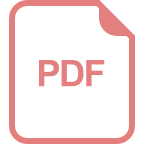
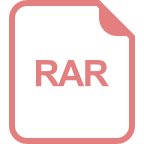
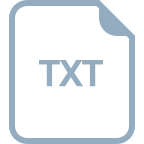
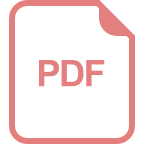
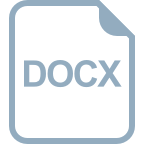
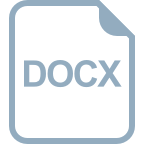
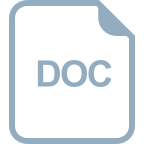
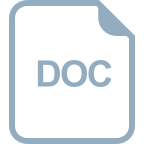
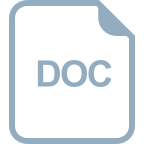
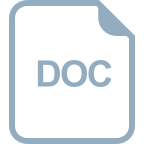
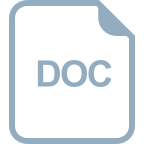