numpy加载csv
时间: 2023-10-21 19:03:32 浏览: 30
你可以使用Python中的numpy库来加载CSV文件。下面是一个简单的例子:
```python
import numpy as np
# 读取CSV文件
data = np.loadtxt("path/to/file.csv", delimiter=",")
# 打印数据
print(data)
```
在上面的例子中,`loadtxt`函数用于从CSV文件中读取数据,`delimiter`参数用于指定列之间的分隔符。你需要替换`path/to/file.csv`为你实际的CSV文件路径。注意,这只适用于CSV文件中的所有列都是数字的情况。如果你的CSV文件中包含字符串或其他非数字类型,你需要使用pandas库来加载它。
相关问题
numpy读取csv某一列
可以使用numpy的loadtxt函数来读取csv文件中的某一列数据。首先需要将csv文件加载为numpy数组,然后使用数组的索引来获取所需的列。
以下是一个读取csv文件某一列数据的示例代码:
```python
import numpy as np
# 加载csv文件为numpy数组
data = np.loadtxt('data.csv', delimiter=',', skiprows=1)
# 获取第二列数据
col2 = data[:, 1]
# 输出第二列数据
print(col2)
```
在上面的代码中,`loadtxt`函数用于加载csv文件。`delimiter`参数用于指定分隔符,`skiprows`参数用于跳过文件的第一行标题。然后使用`[:, 1]`来获取第二列数据,其中`:`表示选择所有行,`1`表示选择第二列。最后,输出所需的列数据即可。
numpy怎么读取csv文件
要使用NumPy读取csv文件,可以使用loadtxt()函数。以下是一个简单的例子:
```python
import numpy as np
data = np.loadtxt('file.csv', delimiter=',', skiprows=1)
print(data)
```
在这个例子中,我们首先导入NumPy库。然后,我们使用loadtxt()函数加载名为“file.csv”的文件,并将分隔符设置为逗号。skiprows=1参数告诉函数跳过文件的第一行(通常是标题行)。最后,我们将结果存储在名为data的变量中,并打印输出。
相关推荐
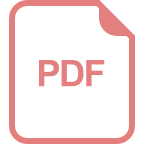
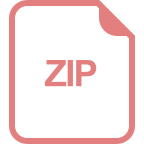
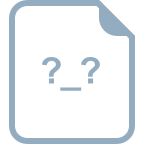












