用C++和Easyx在VC中设置两个小球,在窗口中进行运动 要求:当二者进行碰撞时,随机方向进行弹开,运动小球不能出窗口
时间: 2024-02-19 22:58:36 浏览: 22
好的,这是一个使用 C++ 和 EasyX 图形库在 VC 中实现的小游戏,以下是实现代码:
```C++
#include <graphics.h>
#include <stdio.h#include <stdlib.h>
#include <time.h>
#include <math.h>
// 窗口宽度和高度
const int WIDTH = 800;
const int HEIGHT = 600;
// 定义小球类
class Ball {
public:
Ball(int x, int y, int radius, COLORREF color) {
this->x = x;
this->y = y;
this->radius = radius;
this->color = color;
this->speed_x = rand() % 2 == 0 ? -5 : 5; // 随机初始化 x 方向速度
this->speed_y = rand() % 2 == 0 ? -5 : 5; // 随机初始化 y 方向速度
}
// 移动小球
void move() {
this->x += this->speed_x;
this->y += this->speed_y;
}
// 检测小球是否碰撞到窗口边界
void check_boundary_collision() {
if (this->x - this->radius < 0 || this->x + this->radius > WIDTH) {
this->speed_x = -this->speed_x;
}
if (this->y - this->radius < 0 || this->y + this->radius > HEIGHT) {
this->speed_y = -this->speed_y;
}
}
// 检测小球是否碰撞到另一个小球
void check_ball_collision(Ball& other_ball) {
int distance = sqrt(pow(this->x - other_ball.x, 2) + pow(this->y - other_ball.y, 2));
if (distance < this->radius + other_ball.radius) {
// 随机初始化碰撞后的速度方向
this->speed_x = rand() % 2 == 0 ? -5 : 5;
this->speed_y = rand() % 2 == 0 ? -5 : 5;
other_ball.speed_x = rand() % 2 == 0 ? -5 : 5;
other_ball.speed_y = rand() % 2 == 0 ? -5 : 5;
}
}
// 绘制小球
void draw() {
setfillcolor(this->color);
setlinecolor(this->color);
fillcircle(this->x, this->y, this->radius);
}
private:
int x, y, radius, speed_x, speed_y;
COLORREF color;
};
int main() {
// 初始化随机数种子
srand(time(NULL));
// 创建窗口
initgraph(WIDTH, HEIGHT);
setbkcolor(WHITE);
// 创建两个小球
Ball ball1(50, 50, 30, RED);
Ball ball2(200, 200, 50, BLUE);
// 游戏循环
while (true) {
// 移动小球
ball1.move();
ball2.move();
// 检测小球是否碰撞到窗口边界
ball1.check_boundary_collision();
ball2.check_boundary_collision();
// 检测小球是否碰撞到另一个小球
ball1.check_ball_collision(ball2);
// 绘制窗口和小球
cleardevice();
ball1.draw();
ball2.draw();
Sleep(10);
}
// 关闭窗口
closegraph();
return 0;
}
```
当你运行这个程序时,会显示出一个窗口,里面有两个小球在随机运动,当两个小球碰撞时,会随机弹开。
相关推荐
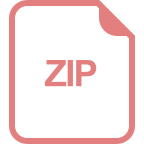














