用C语言实现静态链表的初始化,插入和删除
时间: 2024-09-13 21:08:13 浏览: 57
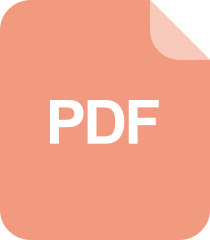
C语言实现静态链表

在C语言中,静态链表通常使用数组而不是动态内存分配来存储元素,因为其大小在编译时就已经确定。下面是一个简单的例子,展示如何初始化、插入和删除静态链表节点:
```c
#include <stdio.h>
// 链表节点结构体
typedef struct {
int data;
struct Node *next; // 指向下一个节点的指针
} Node;
// 初始化静态链表
void initStaticList(Node list[], int size) {
for (int i = 0; i < size; i++) {
list[i].data = 0; // 初始值可以自定义
list[i].next = &list[(i + 1) % size]; // 循环链接,最后一个节点连接到第一个
}
list[0].next = &list[0]; // 将循环链表首尾相连
}
// 插入节点
void insertNode(Node list[], int index, int value) {
if (index >= 0 && index < sizeof(list) / sizeof(list[0])) {
Node newNode = {value, NULL};
Node *current = list;
for (int i = 0; i < index; i++, current = current->next) {
newNode.next = current->next;
}
current->next = &newNode;
} else {
printf("Invalid index.\n");
}
}
// 删除节点(这里假设链表元素不可重复)
void deleteNode(Node list[], int index) {
if (index >= 0 && index < sizeof(list) / sizeof(list[0])) {
Node *prev = NULL, *current = list;
for (int i = 0; i < index; i++, prev = current, current = current->next) {}
prev->next = current->next;
} else {
printf("Invalid index.\n");
}
}
// 打印链表
void printList(Node list[]) {
for (Node *node = list; node != NULL; node = node->next) {
printf("%d ", node->data);
}
printf("\n");
}
int main() {
Node staticList[5] = {0}; // 创建一个包含5个节点的静态链表
initStaticList(staticList, 5);
insertNode(staticList, 2, 10); // 在索引2处插入值10
deleteNode(staticList, 1); // 删除索引1处的节点
printList(staticList); // 输出链表内容
return 0;
}
```
阅读全文
相关推荐
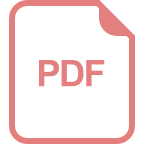
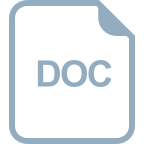
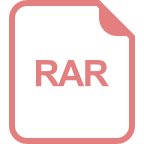
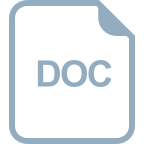
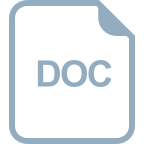

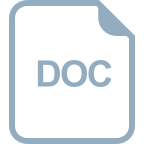
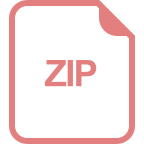
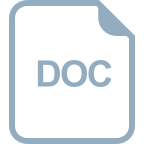


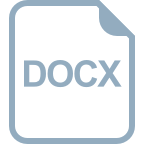
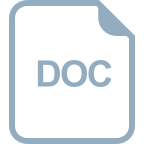
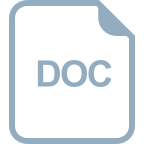
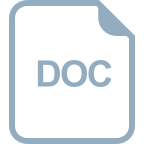
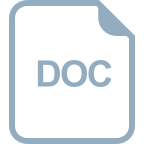


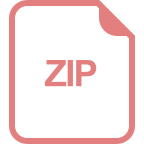