我想在unity 的rawimage上画一条折线图
时间: 2023-01-11 08:48:09 浏览: 189
首先,你需要创建一个新的脚本,然后在脚本中定义一个绘制折线图的函数。这个函数应该接受一个数组作为输入,这个数组包含了折线图中各个点的值。然后,你可以使用 Unity 的绘图 API 在 RawImage 上绘制折线图。
具体来说,你可以使用 Graphics.DrawTexture() 函数在 RawImage 上绘制一张图片。这张图片的像素数据就是你要绘制的折线图的像素数据。你可以使用 Texture2D.SetPixels() 函数为这张图片设置像素数据。然后使用 Texture2D.Apply() 函数将像素数据应用到图片上。
最后,你需要在 Update 函数中调用你定义的绘制折线图的函数,这样就能够在每一帧都绘制折线图了。
如果你不熟悉 Unity 的绘图 API,可以先阅读一下 Unity 官方文档中关于这些函数的介绍,然后再尝试实现你的功能。
相关问题
我想在unity 的rawimage上画一条折线图 请写出完整脚本
在 Unity 中,你可以使用 Unity 的绘图 API(Graphics.DrawTexture)来在 RawImage 上绘制折线图。这是一个完整的脚本示例:
```
using UnityEngine;
using UnityEngine.UI;
public class LineGraph : MonoBehaviour
{
// 要在其上绘制折线图的 RawImage
public RawImage image;
// 折线图的数据点
public Vector2[] points;
// 折线图的颜色
public Color lineColor;
void Start()
{
// 创建一个新的纹理,它的大小与 RawImage 相同
Texture2D texture = new Texture2D((int)image.rectTransform.rect.width, (int)image.rectTransform.rect.height);
// 在纹理上绘制折线图
DrawLineGraph(texture, points, lineColor);
// 将纹理赋给 RawImage
image.texture = texture;
}
void DrawLineGraph(Texture2D texture, Vector2[] points, Color color)
{
// 设置纹理的像素
for (int i = 0; i < points.Length - 1; i++)
{
DrawLine(texture, points[i], points[i + 1], color);
}
}
void DrawLine(Texture2D texture, Vector2 p1, Vector2 p2, Color color)
{
// 计算折线的斜率
float slope = (p2.y - p1.y) / (p2.x - p1.x);
// 如果斜率不存在(即折线为水平或竖直线),则特别处理
if (float.IsInfinity(slope))
{
int x1 = (int)p1.x;
int x2 = (int)p2.x;
int y = (int)p1.y;
if (x1 > x2)
{
int temp = x1;
x1 = x2;
x2 = temp;
}
for (int x = x1; x <= x2; x++)
{
texture.SetPixel(x, y, color);
}
}
else
{
// 计算斜率的截距
float intercept = p1.y - slope * p1.x;
// 将折线拆
我想在unity的rawimage上绘制一条折线图,请写出完整的脚本
好的,我们可以在 Unity 中使用 LineRenderer 组件来绘制折线图。首先,你需要在你的场景中创建一个 GameObject,并为其添加 LineRenderer 组件。然后,你可以使用 LineRenderer 的 SetPositions 方法来设置折线图的点的位置。这些点的位置可以通过脚本来动态设置。
具体的脚本代码如下:
```
using UnityEngine;
public class LineRendererExample : MonoBehaviour
{
// 声明一个 LineRenderer 组件
LineRenderer lineRenderer;
void Start()
{
// 获取 LineRenderer 组件
lineRenderer = GetComponent<LineRenderer>();
// 创建一个新的 Vector3 数组,用于保存折线图的点的位置
Vector3[] points = new Vector3[5];
// 设置每个点的位置
points[0] = new Vector3(-2, 0, 0);
points[1] = new Vector3(-1, 1, 0);
points[2] = new Vector3(0, 0, 0);
points[3] = new Vector3(1, -1, 0);
points[4] = new Vector3(2, 0, 0);
// 使用 SetPositions 方法来设置折线图的点的位置
lineRenderer.SetPositions(points);
}
}
```
上面的脚本中,我们在 Start 函数中创建了一个 Vector3 数组,并为每个点设置了位置。然后使用 LineRenderer 的 SetPositions 方法来设置折线图的点的位置。
我希望这个脚本能帮到你。如果你还有其他问题,可以继续问我。
阅读全文
相关推荐









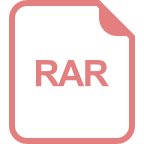



