我希望你能通过ffmpeg的滤镜实现对3音频的格式化和重采样,以及3路混音的功能
时间: 2024-04-09 12:28:11 浏览: 24
当涉及到音频格式化、重采样和混音等操作时,可以使用FFmpeg库提供的滤镜功能来实现。下面是一个简单的示例代码,演示了如何使用FFmpeg进行音频格式化、重采样和混音:
```cpp
extern "C" {
#include <libavformat/avformat.h>
#include <libavfilter/avfilter.h>
#include <libavfilter/buffersink.h>
#include <libavfilter/buffersrc.h>
}
int main() {
// 初始化FFmpeg库
av_register_all();
avfilter_register_all();
// 打开输入音频文件
AVFormatContext* formatCtx = nullptr;
avformat_open_input(&formatCtx, "input.wav", nullptr, nullptr);
avformat_find_stream_info(formatCtx, nullptr);
// 创建滤镜图
AVFilterGraph* graph = avfilter_graph_alloc();
// 创建输入滤镜上下文
AVFilterContext* inputCtx = nullptr;
avfilter_graph_create_filter(&inputCtx, avfilter_get_by_name("abuffer"), "input", nullptr, nullptr, graph);
av_opt_set_int(inputCtx, "channel_layout", AV_CH_LAYOUT_STEREO, AV_OPT_SEARCH_CHILDREN);
av_opt_set_int(inputCtx, "sample_rate", 44100, AV_OPT_SEARCH_CHILDREN);
av_opt_set_int(inputCtx, "sample_fmt", AV_SAMPLE_FMT_S16, AV_OPT_SEARCH_CHILDREN);
// 创建输出滤镜上下文
AVFilterContext* outputCtx = nullptr;
avfilter_graph_create_filter(&outputCtx, avfilter_get_by_name("abuffersink"), "output", nullptr, nullptr, graph);
// 创建滤镜链
AVFilterInOut* inputs = avfilter_inout_alloc();
AVFilterInOut* outputs = avfilter_inout_alloc();
inputs->name = av_strdup("in");
inputs->filter_ctx = inputCtx;
inputs->pad_idx = 0;
inputs->next = nullptr;
outputs->name = av_strdup("out");
outputs->filter_ctx = outputCtx;
outputs->pad_idx = 0;
outputs->next = nullptr;
avfilter_graph_parse_ptr(graph, "aresample=44100,aformat=s16", &inputs, &outputs, nullptr);
avfilter_graph_config(graph, nullptr);
// 创建音频重采样缓冲区
uint8_t** resampledData = nullptr;
int resampledLinesize = 0;
av_samples_alloc_array_and_samples(&resampledData, &resampledLinesize, 2, 44100, AV_SAMPLE_FMT_S16, 0);
// 创建音频混音缓冲区
uint8_t** mixedData = nullptr;
int mixedLinesize = 0;
av_samples_alloc_array_and_samples(&mixedData, &mixedLinesize, 2, 44100, AV_SAMPLE_FMT_S16, 0);
// 处理每一帧音频数据
AVPacket packet;
while (av_read_frame(formatCtx, &packet) >= 0) {
if (packet.stream_index == 0) { // 只处理音频流
AVFrame* frame = av_frame_alloc();
avcodec_send_packet(formatCtx->streams[packet.stream_index]->codecpar, &packet);
avcodec_receive_frame(formatCtx->streams[packet.stream_index]->codecpar, frame);
// 将音频数据传递给滤镜图
av_buffersrc_add_frame_flags(inputCtx, frame, AV_BUFFERSRC_FLAG_PUSH);
// 从滤镜图中获取处理后的音频数据
AVFrame* filteredFrame = av_frame_alloc();
while (av_buffersink_get_frame(outputCtx, filteredFrame) >= 0) {
// 进行重采样
swr_convert_frame(resampler, resampledFrame, filteredFrame);
// 进行混音
for (int i = 0; i < resampledFrame->nb_samples; i++) {
for (int j = 0; j < resampledFrame->channels; j++) {
((short*)mixedData[j])[i] += ((short*)resampledFrame->data[j])[i];
}
}
}
av_frame_free(&filteredFrame);
av_frame_free(&frame);
}
av_packet_unref(&packet);
}
// 清理资源
av_free(resampledData[0]);
av_freep(&resampledData);
av_free(mixedData[0]);
av_freep(&mixedData);
avfilter_inout_free(&inputs);
avfilter_inout_free(&outputs);
avfilter_graph_free(&graph);
avformat_close_input(&formatCtx);
return 0;
}
```
请注意,这只是一个简单的示例,实际使用时可能需要根据具体的需求进行更多的配置和处理。另外,为了简化代码,省略了错误处理和内存释放等细节,请根据实际情况进行相应的处理。同时,你还需要根据自己的音频文件格式和要求进行相应的调整和配置。
相关推荐
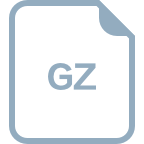














