STM32F103固件库建立tcp客户端,在建立数据接收回调函数时将数据一个一个写入环形缓冲区,并在主函数从缓冲区读出数据,并发送出去,代码
时间: 2024-03-10 20:48:56 浏览: 35
以下是一个简单的示例代码,用于建立TCP客户端并将数据写入环形缓冲区:
```c
#include "stm32f10x.h"
#include "lwip/opt.h"
#include "lwip/arch.h"
#include "lwip/api.h"
#define TCP_CLIENT_BUFFER_SIZE 1024
static char tcp_client_buffer[TCP_CLIENT_BUFFER_SIZE];
static u16_t tcp_client_write_index = 0;
static u16_t tcp_client_read_index = 0;
static struct netconn *tcp_client_conn = NULL;
void tcp_client_data_receive_callback(void *arg, struct netconn *conn, enum netconn_evt evt, u16_t len)
{
if (evt == NETCONN_EVT_RCVMINUS) {
return;
}
char *recv_buf = malloc(len);
if (recv_buf == NULL) {
return;
}
struct netbuf *recv_netbuf = NULL;
if (netconn_recv(conn, &recv_netbuf) == ERR_OK) {
void *data;
u16_t recv_len;
while (netbuf_data(recv_netbuf, &data, &recv_len) == ERR_OK) {
memcpy(recv_buf, data, recv_len);
recv_buf += recv_len;
}
netbuf_delete(recv_netbuf);
// write received data to circular buffer
for (u16_t i = 0; i < len; i++) {
tcp_client_buffer[tcp_client_write_index] = *(recv_buf - len + i);
tcp_client_write_index = (tcp_client_write_index + 1) % TCP_CLIENT_BUFFER_SIZE;
}
}
free(recv_buf);
}
void tcp_client_send_data(void)
{
if (tcp_client_conn == NULL) {
return;
}
while (tcp_client_write_index != tcp_client_read_index) {
u16_t send_len = (tcp_client_write_index > tcp_client_read_index) ?
(tcp_client_write_index - tcp_client_read_index) :
(TCP_CLIENT_BUFFER_SIZE - tcp_client_read_index);
struct netbuf *send_netbuf = netbuf_new();
if (send_netbuf == NULL) {
return;
}
char *send_buf = malloc(send_len);
if (send_buf == NULL) {
netbuf_delete(send_netbuf);
return;
}
// read data from circular buffer
for (u16_t i = 0; i < send_len; i++) {
*(send_buf + i) = tcp_client_buffer[tcp_client_read_index];
tcp_client_read_index = (tcp_client_read_index + 1) % TCP_CLIENT_BUFFER_SIZE;
}
netbuf_ref(send_netbuf, send_buf, send_len);
if (netconn_send(tcp_client_conn, send_netbuf) != ERR_OK) {
netbuf_delete(send_netbuf);
free(send_buf);
return;
}
netbuf_delete(send_netbuf);
free(send_buf);
}
}
void tcp_client_init(void)
{
tcp_client_conn = netconn_new(NETCONN_TCP);
if (tcp_client_conn == NULL) {
return;
}
ip_addr_t server_ipaddr;
IP4_ADDR(&server_ipaddr, 192, 168, 1, 100);
err_t err = netconn_connect(tcp_client_conn, &server_ipaddr, 5000);
if (err != ERR_OK) {
netconn_delete(tcp_client_conn);
tcp_client_conn = NULL;
return;
}
netconn_set_recvtimeout(tcp_client_conn, 10);
netconn_set_nonblocking(tcp_client_conn, 1);
netconn_set_recv_callback(tcp_client_conn, tcp_client_data_receive_callback);
}
int main(void)
{
tcp_client_init();
while (1) {
tcp_client_send_data();
// do other things
}
return 0;
}
```
这个示例代码中使用了lwIP协议栈,通过netconn API建立TCP客户端连接,并在数据接收回调函数中将接收到的数据写入环形缓冲区。发送数据时,从环形缓冲区读取数据并发送到服务器。注意,这个示例代码只是一个简单的演示,仅供参考,具体实现需要根据你的具体需求进行修改。
相关推荐
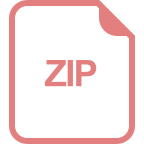
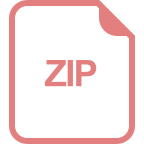
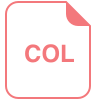













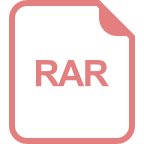