java三角形三个顶点的颜色分别为红绿蓝,使用有效边表算法填充三角形
时间: 2023-06-18 11:03:28 浏览: 100
以下是使用有效边表算法填充三角形的Java代码:
```java
import java.awt.Color;
import java.awt.Graphics;
import java.awt.Point;
import java.util.ArrayList;
import java.util.Collections;
import java.util.Comparator;
import javax.swing.JFrame;
import javax.swing.JPanel;
public class TriangleFill extends JPanel {
private static final long serialVersionUID = 1L;
private Point[] vertices = { new Point(100, 100), new Point(150, 200), new Point(200, 150) };
private Color[] colors = { Color.RED, Color.GREEN, Color.BLUE };
private ArrayList<Edge> edges = new ArrayList<Edge>();
private ArrayList<Edge> activeEdges = new ArrayList<Edge>();
public TriangleFill() {
// Initialize edges
for (int i = 0; i < 3; i++) {
Point v1 = vertices[i];
Point v2 = vertices[(i + 1) % 3];
if (v1.y > v2.y) {
Point temp = v1;
v1 = v2;
v2 = temp;
}
Color c1 = colors[i];
Color c2 = colors[(i + 1) % 3];
int dx = v2.x - v1.x;
int dy = v2.y - v1.y;
double slope = (dx == 0) ? 0 : (double) dy / dx;
Edge edge = new Edge(v1.y, v2.y, v1.x, slope, c1, c2);
edges.add(edge);
}
// Sort edges by ymin
Collections.sort(edges, new Comparator<Edge>() {
public int compare(Edge e1, Edge e2) {
return e1.ymin - e2.ymin;
}
});
}
public void paintComponent(Graphics g) {
super.paintComponent(g);
// Draw vertices
for (int i = 0; i < 3; i++) {
g.setColor(colors[i]);
g.fillOval(vertices[i].x - 2, vertices[i].y - 2, 4, 4);
}
// Fill triangle
int ymin = edges.get(0).ymin;
int ymax = edges.get(edges.size() - 1).ymax;
for (int y = ymin; y <= ymax; y++) {
// Update active edges
for (int i = 0; i < activeEdges.size(); i++) {
Edge activeEdge = activeEdges.get(i);
if (y == activeEdge.ymax) {
activeEdges.remove(i);
i--;
} else {
activeEdge.x += activeEdge.slope;
activeEdge.color = interpolateColor(activeEdge.c1, activeEdge.c2, (activeEdge.x - activeEdge.xmin) / (activeEdge.xmax - activeEdge.xmin));
}
}
// Add new edges
while (!edges.isEmpty() && edges.get(0).ymin == y) {
activeEdges.add(edges.get(0));
edges.remove(0);
}
// Sort active edges by x
Collections.sort(activeEdges, new Comparator<Edge>() {
public int compare(Edge e1, Edge e2) {
return (int) (e1.x - e2.x);
}
});
// Fill scanline
for (int i = 0; i < activeEdges.size(); i += 2) {
Edge leftEdge = activeEdges.get(i);
Edge rightEdge = activeEdges.get(i + 1);
g.setColor(interpolateColor(leftEdge.color, rightEdge.color, 0.5));
g.drawLine((int) leftEdge.x, y, (int) rightEdge.x, y);
}
}
}
private Color interpolateColor(Color c1, Color c2, double t) {
int r = (int) ((1 - t) * c1.getRed() + t * c2.getRed());
int g = (int) ((1 - t) * c1.getGreen() + t * c2.getGreen());
int b = (int) ((1 - t) * c1.getBlue() + t * c2.getBlue());
return new Color(r, g, b);
}
public static void main(String[] args) {
JFrame frame = new JFrame("Triangle Fill");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setSize(300, 300);
frame.add(new TriangleFill());
frame.setVisible(true);
}
private class Edge {
public int ymin;
public int ymax;
public double x;
public double slope;
public Color c1;
public Color c2;
public Color color;
public int xmin;
public int xmax;
public Edge(int ymin, int ymax, int xmin, double slope, Color c1, Color c2) {
this.ymin = ymin;
this.ymax = ymax;
this.x = xmin;
this.slope = slope;
this.c1 = c1;
this.c2 = c2;
this.color = c1;
this.xmin = xmin;
this.xmax = (int) Math.round(xmin + (ymax - ymin) / slope);
}
}
}
```
这个程序会绘制一个三角形,并使用有效边表算法填充三角形。在程序运行时,你会看到三角形被填充为红绿蓝相间的颜色。
阅读全文
相关推荐






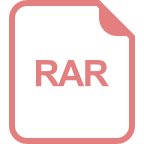




