#include <stdio.h> #include <stdlib.h> #include <unistd.h> #include <string.h> #include <fcntl.h> #include <sys/ioctl.h> #include <linux/can.h> #include <linux/can/raw.h> #define CAN_INTERFACE "can0" // CAN接口名称 #define CAN_ID 0x123 // CAN ID int main(void) { int s; // socket描述符 struct sockaddr_can addr; struct ifreq ifr; struct can_frame frame; int nbytes; // 创建socket s = socket(PF_CAN, SOCK_RAW, CAN_RAW); if(s < 0) { perror("socket PF_CAN failed"); return 1; } // 设置CAN接口 strcpy(ifr.ifr_name, CAN_INTERFACE); ioctl(s, SIOCGIFINDEX, &ifr); addr.can_family = PF_CAN; addr.can_ifindex = ifr.ifr_ifindex; bind(s, (struct sockaddr *)&addr, sizeof(addr)); // 发送CAN数据 frame.can_id = CAN_ID; frame.can_dlc = 2; frame.data[0] = 0x01; frame.data[1] = 0x02; nbytes = write(s, &frame, sizeof(struct can_frame)); if(nbytes != sizeof(struct can_frame)) { perror("write failed"); close(s); return 1; } // 接收CAN数据 nbytes = read(s, &frame, sizeof(struct can_frame)); if(nbytes < 0) { perror("read failed"); close(s); return 1; } printf("can_id = 0x%X, can_dlc = %d, data = %02X %02X\n", frame.can_id, frame.can_dlc, frame.data[0], frame.data[1]); close(s); return 0; }这个代码gcc编译不过,重新写一下呢?
时间: 2024-03-02 08:49:50 浏览: 22
这段代码的问题在于缺少头文件的引用,需要添加以下头文件:
```c
#include <sys/types.h>
#include <sys/socket.h>
#include <net/if.h>
#include <netinet/in.h>
```
另外,建议在使用 ioctl 函数时加上错误处理,修改后的代码如下:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <string.h>
#include <fcntl.h>
#include <sys/ioctl.h>
#include <sys/types.h>
#include <sys/socket.h>
#include <net/if.h>
#include <netinet/in.h>
#include <linux/can.h>
#include <linux/can/raw.h>
#define CAN_INTERFACE "can0" // CAN接口名称
#define CAN_ID 0x123 // CAN ID
int main(void) {
int s; // socket描述符
struct sockaddr_can addr;
struct ifreq ifr;
struct can_frame frame;
int nbytes;
// 创建socket
s = socket(PF_CAN, SOCK_RAW, CAN_RAW);
if(s < 0) {
perror("socket PF_CAN failed");
return 1;
}
// 设置CAN接口
strcpy(ifr.ifr_name, CAN_INTERFACE);
if(ioctl(s, SIOCGIFINDEX, &ifr) < 0) {
perror("ioctl failed");
close(s);
return 1;
}
addr.can_family = PF_CAN;
addr.can_ifindex = ifr.ifr_ifindex;
if(bind(s, (struct sockaddr *)&addr, sizeof(addr)) < 0) {
perror("bind failed");
close(s);
return 1;
}
// 发送CAN数据
frame.can_id = CAN_ID;
frame.can_dlc = 2;
frame.data[0] = 0x01;
frame.data[1] = 0x02;
nbytes = write(s, &frame, sizeof(struct can_frame));
if(nbytes != sizeof(struct can_frame)) {
perror("write failed");
close(s);
return 1;
}
// 接收CAN数据
nbytes = read(s, &frame, sizeof(struct can_frame));
if(nbytes < 0) {
perror("read failed");
close(s);
return 1;
}
printf("can_id = 0x%X, can_dlc = %d, data = %02X %02X\n",
frame.can_id, frame.can_dlc, frame.data[0], frame.data[1]);
close(s);
return 0;
}
```
相关推荐
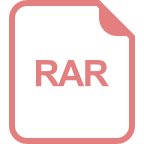
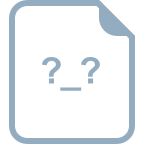
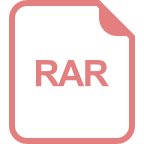















