python中怎么安装imread库、inwrite库和imshow库
时间: 2024-05-01 11:20:19 浏览: 11
在Python中,imread、inwrite和imshow是OpenCV库中的函数,用于读取、写入和显示图像。
要安装OpenCV和其依赖库,请执行以下步骤:
1. 打开命令行终端或Anaconda Prompt。
2. 输入以下命令安装OpenCV:
```
pip install opencv-python
```
或者:
```
conda install -c conda-forge opencv
```
3. 安装完成后,即可在Python中调用imread、inwrite和imshow函数。
例如,以下是使用OpenCV在Python中读取、修改和显示图像的示例代码:
```
import cv2
# 读取图像
img = cv2.imread('image.jpg')
# 修改图像
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
# 显示图像
cv2.imshow('image', gray)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
相关问题
使用python的opencv库自制TSPLIB,选点代码
下面是使用Python的OpenCV库自制TSPLIB的选点代码:
```python
import cv2
import numpy as np
import random
# 读取图片
img = cv2.imread('your_image_path.jpg')
# 转换为灰度图
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
# Canny边缘检测
edges = cv2.Canny(gray, 50, 150, apertureSize=3)
# 霍夫直线变换,检测直线
lines = cv2.HoughLines(edges, 1, np.pi/180, 200)
# 绘制直线
for line in lines:
rho, theta = line[0]
a = np.cos(theta)
b = np.sin(theta)
x0 = a*rho
y0 = b*rho
x1 = int(x0 + 1000*(-b))
y1 = int(y0 + 1000*(a))
x2 = int(x0 - 1000*(-b))
y2 = int(y0 - 1000*(a))
cv2.line(img, (x1, y1), (x2, y2), (0, 0, 255), 2)
# 随机选取点
points = []
for i in range(10):
x = random.randint(0, img.shape[1])
y = random.randint(0, img.shape[0])
points.append((x, y))
cv2.circle(img, (x, y), 5, (0, 255, 0), -1)
# 保存选点结果
with open('your_tsp_file.tsp', 'w') as f:
f.write('NAME: your_tsp_file\n')
f.write('TYPE: TSP\n')
f.write(f'DIMENSION: {len(points)}\n')
f.write('EDGE_WEIGHT_TYPE: EUC_2D\n')
f.write('NODE_COORD_SECTION\n')
for i, point in enumerate(points):
f.write(f'{i+1} {point[0]} {point[1]}\n')
f.write('EOF\n')
# 显示结果
cv2.imshow('img', img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
这段代码使用Canny边缘检测和霍夫直线变换检测图片中的直线,并随机选取10个点作为TSP问题的节点,然后将节点坐标保存到文件中。您可以根据需要修改代码以适应不同的图片和节点数量。
using python how to write a code for detecting cat in a picture
As an AI language model, I do not have the ability to write and execute code. However, I can provide you with a sample code in Python for detecting cats in an image using OpenCV library and a pre-trained Haar classifier.
```
import cv2
# Load the pre-trained Haar classifier for cat detection
cat_cascade = cv2.CascadeClassifier('haarcascade_frontalcatface.xml')
# Load the image to detect cats
img = cv2.imread('cat.jpg')
# Convert the image to grayscale
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
# Detect cats in the image
cats = cat_cascade.detectMultiScale(gray, scaleFactor=1.1, minNeighbors=5)
# Draw rectangles around the detected cats
for (x,y,w,h) in cats:
cv2.rectangle(img, (x,y), (x+w,y+h), (0,255,0), 2)
# Display the image with detected cats
cv2.imshow('Cat Detection', img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
In this code, we first load the pre-trained Haar classifier for cat detection using `CascadeClassifier` function from OpenCV library. We then load the image to detect cats and convert it to grayscale using `cvtColor` function. We use `detectMultiScale` function to detect cats in the grayscale image and draw rectangles around the detected cats using `rectangle` function. Finally, we display the image with detected cats using `imshow` function and wait for the user to press any key to exit the display window.
相关推荐
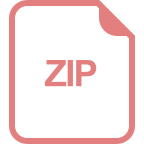
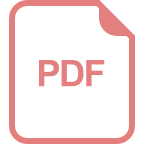
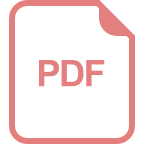













