用javafx模拟汽车上的雨刷器运行动画
时间: 2023-12-03 16:46:20 浏览: 135
好的,我可以为您提供一些简单的代码示例,帮助您开始使用JavaFX创建汽车上的雨刷器动画。
首先,您需要创建一个JavaFX应用程序,并在其中创建一个场景和一个Canvas对象,用于绘制动画。在场景中添加一个Canvas对象,设置其大小并将其添加到场景中:
```java
public class RainWiperAnimation extends Application {
private final int CANVAS_WIDTH = 800;
private final int CANVAS_HEIGHT = 600;
@Override
public void start(Stage primaryStage) {
// Create a canvas object
Canvas canvas = new Canvas(CANVAS_WIDTH, CANVAS_HEIGHT);
// Add the canvas to a pane
Pane root = new Pane(canvas);
// Create a scene
Scene scene = new Scene(root, CANVAS_WIDTH, CANVAS_HEIGHT);
// Add the scene to the primary stage
primaryStage.setScene(scene);
primaryStage.show();
}
}
```
接下来,在Canvas对象上绘制汽车和雨刷器。您可以使用JavaFX的形状和路径API来绘制它们。在这个例子中,我们将绘制一个矩形作为汽车和一个圆形作为雨刷器:
```java
public class RainWiperAnimation extends Application {
private final int CANVAS_WIDTH = 800;
private final int CANVAS_HEIGHT = 600;
@Override
public void start(Stage primaryStage) {
// Create a canvas object
Canvas canvas = new Canvas(CANVAS_WIDTH, CANVAS_HEIGHT);
// Get the graphics context of the canvas
GraphicsContext gc = canvas.getGraphicsContext2D();
// Draw the car
gc.setFill(Color.BLACK);
gc.fillRect(200, 200, 400, 200);
// Draw the wiper
gc.setFill(Color.GRAY);
gc.fillOval(400, 150, 100, 100);
// Add the canvas to a pane
Pane root = new Pane(canvas);
// Create a scene
Scene scene = new Scene(root, CANVAS_WIDTH, CANVAS_HEIGHT);
// Add the scene to the primary stage
primaryStage.setScene(scene);
primaryStage.show();
}
}
```
现在我们已经绘制了汽车和雨刷器,我们需要创建一个动画来模拟雨刷器的运动。我们可以使用JavaFX的动画类来实现这一点。在这个例子中,我们将创建一个Timeline对象,并每秒更新雨刷器的位置:
```java
public class RainWiperAnimation extends Application {
private final int CANVAS_WIDTH = 800;
private final int CANVAS_HEIGHT = 600;
@Override
public void start(Stage primaryStage) {
// Create a canvas object
Canvas canvas = new Canvas(CANVAS_WIDTH, CANVAS_HEIGHT);
// Get the graphics context of the canvas
GraphicsContext gc = canvas.getGraphicsContext2D();
// Draw the car
gc.setFill(Color.BLACK);
gc.fillRect(200, 200, 400, 200);
// Draw the wiper
gc.setFill(Color.GRAY);
gc.fillOval(400, 150, 100, 100);
// Create a timeline for the wiper animation
Timeline timeline = new Timeline(
new KeyFrame(Duration.ZERO, new KeyValue(gc.rotateProperty(), 0)),
new KeyFrame(Duration.seconds(1), new KeyValue(gc.rotateProperty(), 360))
);
// Set the timeline to repeat indefinitely
timeline.setCycleCount(Timeline.INDEFINITE);
// Play the timeline
timeline.play();
// Add the canvas to a pane
Pane root = new Pane(canvas);
// Create a scene
Scene scene = new Scene(root, CANVAS_WIDTH, CANVAS_HEIGHT);
// Add the scene to the primary stage
primaryStage.setScene(scene);
primaryStage.show();
}
}
```
现在我们已经创建了一个动画,并将其应用于雨刷器。当应用程序运行时,您将看到雨刷器在汽车上来回移动。
这只是一个简单的示例,您可以根据需要根据实际情况对其进行修改和扩展。
阅读全文
相关推荐
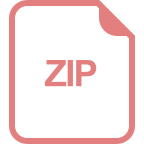
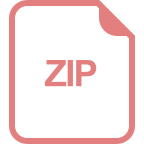
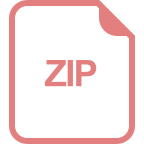



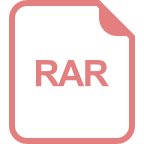
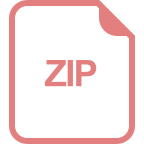










