JavaFX代码实现汽车雨刷不同挡位左右摇摆
时间: 2023-12-10 09:58:08 浏览: 148
以下是一个简单的JavaFX代码示例,可以实现汽车雨刷不同挡位左右摇摆:
```java
import javafx.animation.*;
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.layout.Pane;
import javafx.scene.paint.Color;
import javafx.scene.shape.Line;
import javafx.stage.Stage;
import javafx.util.Duration;
public class CarWiper extends Application {
private final static int WIDTH = 400;
private final static int HEIGHT = 400;
private Line leftWiper;
private Line rightWiper;
@Override
public void start(Stage primaryStage) throws Exception {
Pane root = new Pane();
// 左雨刷
leftWiper = new Line(0, HEIGHT / 2, 0, HEIGHT / 2 - 30);
leftWiper.setStrokeWidth(3);
leftWiper.setStroke(Color.BLACK);
root.getChildren().add(leftWiper);
// 右雨刷
rightWiper = new Line(WIDTH, HEIGHT / 2, WIDTH, HEIGHT / 2 - 30);
rightWiper.setStrokeWidth(3);
rightWiper.setStroke(Color.BLACK);
root.getChildren().add(rightWiper);
Scene scene = new Scene(root, WIDTH, HEIGHT);
primaryStage.setScene(scene);
primaryStage.show();
// 模拟不同挡位
int speed = 1; // 默认挡位
switch (speed) {
case 1:
// 左右交替
Timeline timeline = new Timeline(
new KeyFrame(Duration.ZERO, new KeyValue(leftWiper.startXProperty(), 0), new KeyValue(leftWiper.startYProperty(), HEIGHT / 2), new KeyValue(leftWiper.endXProperty(), 0), new KeyValue(leftWiper.endYProperty(), HEIGHT / 2 - 30), new KeyValue(rightWiper.startXProperty(), WIDTH), new KeyValue(rightWiper.startYProperty(), HEIGHT / 2), new KeyValue(rightWiper.endXProperty(), WIDTH), new KeyValue(rightWiper.endYProperty(), HEIGHT / 2 - 30)),
new KeyFrame(Duration.seconds(1), new KeyValue(leftWiper.startXProperty(), 0), new KeyValue(leftWiper.startYProperty(), HEIGHT / 2 - 30), new KeyValue(leftWiper.endXProperty(), 0), new KeyValue(leftWiper.endYProperty(), HEIGHT / 2), new KeyValue(rightWiper.startXProperty(), WIDTH), new KeyValue(rightWiper.startYProperty(), HEIGHT / 2 - 30), new KeyValue(rightWiper.endXProperty(), WIDTH), new KeyValue(rightWiper.endYProperty(), HEIGHT / 2))
);
timeline.setCycleCount(Timeline.INDEFINITE);
timeline.play();
break;
case 2:
// 左右同时
ParallelTransition parallelTransition = new ParallelTransition(
createWiperAnimation(leftWiper),
createWiperAnimation(rightWiper)
);
parallelTransition.setCycleCount(Timeline.INDEFINITE);
parallelTransition.play();
break;
case 3:
// 顺时针
RotateTransition leftRotate = new RotateTransition(Duration.seconds(1), leftWiper);
leftRotate.setByAngle(360);
leftRotate.setCycleCount(Timeline.INDEFINITE);
leftRotate.play();
RotateTransition rightRotate = new RotateTransition(Duration.seconds(1), rightWiper);
rightRotate.setByAngle(360);
rightRotate.setCycleCount(Timeline.INDEFINITE);
rightRotate.play();
break;
default:
break;
}
}
private Animation createWiperAnimation(Line wiper) {
RotateTransition rotate1 = new RotateTransition(Duration.seconds(0.5), wiper);
rotate1.setByAngle(30);
RotateTransition rotate2 = new RotateTransition(Duration.seconds(0.5), wiper);
rotate2.setByAngle(-30);
return new SequentialTransition(rotate1, rotate2);
}
}
```
在上述代码中,我们首先创建了一个`Pane`作为根节点,并在其中添加了两条线段,分别表示左右雨刷。接着,我们根据不同挡位的要求,使用不同的动画来实现左右摇摆的效果:
- 挡位1:左右交替摇摆
- 使用`Timeline`来交替执行左右雨刷的动画
- 挡位2:左右同时摇摆
- 使用`ParallelTransition`来同时执行左右雨刷的动画
- `createWiperAnimation()`方法用于创建单个雨刷的摇摆动画
- 挡位3:顺时针摇摆
- 使用`RotateTransition`来对左右雨刷进行旋转
最后,我们将不同挡位的动画循环执行,以实现汽车雨刷的效果。
阅读全文
相关推荐
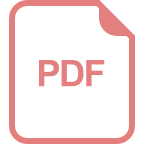
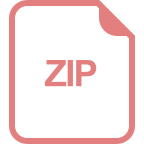
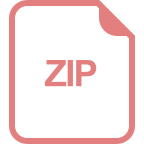

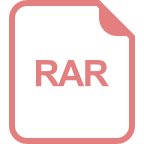
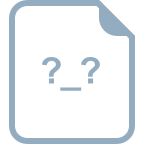
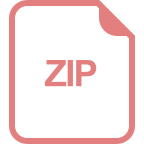
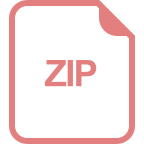
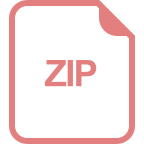
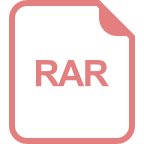
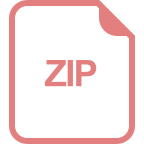
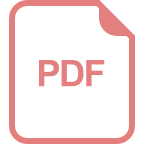
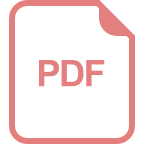
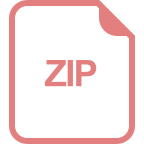