import docx2txt import re # 读取docx文件内容 text = docx2txt.process("example.docx") # 使用正则表达式去重 pattern = r'^(?:(\d+).\s)?―.\n.?[答案]\s*(\w+)\b.*$' # 将去重后的内容写入新的文本文件 with open("example_output.txt", "w", encoding="utf-8") as f: f.write('\n'.join(result.keys()))
时间: 2024-03-04 21:50:36 浏览: 19
这段代码中使用了正则表达式去重,但是没有将匹配到的结果保存下来。可以在代码中添加如下部分,将匹配到的结果保存到一个字典中:
import docx2txt
import re
# 读取docx文件内容
text = docx2txt.process("example.docx")
# 使用正则表达式去重
pattern = r'^(?:(\d+).\s)?―.\n.?[答案]\s*(\w+)\b.*$'
result = {}
for line in text.split('\n'):
match = re.match(pattern, line)
if match:
q_num = match.group(1)
answer = match.group(2)
result[q_num] = answer
# 将去重后的内容写入新的文本文件
with open("example_output.txt", "w", encoding="utf-8") as f:
f.write('\n'.join(result.keys()))
相关问题
补充注释后的代码:import docx2txt import re # 读取docx文件内容 text = docx2txt.process("example.docx") # 使用正则表达式匹配文本中以数字和符号.开头到答案加大写英文字母结束的内容保存为列表 pattern = r'\d+\.\s\w+\s\w+\s\w+\s\w+\s\w+\s\w+' result = list(set(re.findall(pattern, text))) # 去重列表中重复的数据 # 将去重后的内容写入新的文本文件 with open("example_output.txt", "w", encoding="utf-8") as f: f.write('\n'.join(result))
好的,下面是添加注释后的代码:
```
import docx2txt
import re
# 读取docx文件内容
text = docx2txt.process("example.docx")
# 使用正则表达式匹配文本中以数字和符号.开头到答案加大写英文字母结束的内容保存为列表
pattern = r'\d+\.\s\w+\s\w+\s\w+\s\w+\s\w+\s\w+'
result = list(set(re.findall(pattern, text)))
# 去重列表中重复的数据
# 将去重后的内容写入新的文本文件
with open("example_output.txt", "w", encoding="utf-8") as f:
f.write('\n'.join(result))
```
注释应该简明扼要,突出代码的重点和功能,方便其他人理解和维护。
文本文件为空:import docx2txt import re # 读取docx文件内容 text = docx2txt.process("example.docx") # 使用正则表达式匹配文本中以数字和符号.开头到答案加大写英文字母结束的内容保存为列表 pattern = r'\d+\.\s\w+\s\w+\s\w+\s\w+\s\w+\s\w+' result = {} for item in re.findall(pattern, text): result[item] = None # 将去重后的内容写入新的文本文件 with open("example_output.txt", "w", encoding="utf-8") as f: f.write('\n'.join(result.keys()))
可能是因为文本中没有符合正则表达式的内容,导致result字典为空。你可以添加一些代码来判断result字典是否为空,如果为空则输出相应的提示信息。代码如下:
```
import docx2txt
import re
# 读取docx文件内容
text = docx2txt.process("example.docx")
# 使用正则表达式匹配文本中以数字和符号.开头到答案加大写英文字母结束的内容保存为列表
pattern = r'\d+\.\s\w+\s\w+\s\w+\s\w+\s\w+\s\w+'
result = {}
for item in re.findall(pattern, text):
result[item] = None
# 判断result字典是否为空
if not result:
print("没有匹配到符合条件的内容!")
else:
# 将去重后的内容写入新的文本文件
with open("example_output.txt", "w", encoding="utf-8") as f:
f.write('\n'.join(result.keys()))
```
这样当result字典为空时,程序会输出"没有匹配到符合条件的内容!",提醒用户文件中没有符合条件的内容。
相关推荐
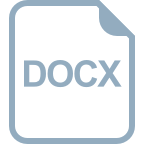
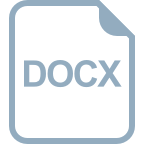
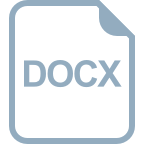













