# include<iostream> using namespace std; class Complex { private: double real, image; public: Complex(double rel = 0, double img = 0) { real = rel; image = img; } void display() { cout << "(" << real; if (image > 0) cout << "+" << image << "*i)"; else if (image < 0) cout << image << "*i)"; else cout << ")"; } friend Complex operator -(Complex& c1, Complex& c2); friend Complex operator /(Complex& c1, Complex& c2); }; Complex operator -(Complex& c1, Complex& c2) { Complex temp; temp.real = c1.real - c2.real; temp.image = c1.image - c2.image; return temp; } Complex operator /(Complex& c1, Complex& c2) { Complex FFF; FFF.real = c1.real / c2.real; FFF.image = c2.image / c2.image; return FFF; } ; int main() { Complex c1(10, 9), c2(3, -4), c3; c3 = c1 / 5; //输出除的结果 c1.display(); cout << "/"; cout << 5; cout << "="; c3.display(); cout << endl; }严重性 代码 说明 项目 文件 行 禁止显示状态 错误 C2679 二元“/”: 没有找到接受“int”类型的右操作数的运算符(或没有可接受的转换) lab6 D:\C++\lab6\lab6\源.cpp 44
时间: 2024-04-04 12:31:15 浏览: 17
这是一个C++的程序,定义了一个复数类Complex,包含实部和虚部,以及显示函数。程序中定义了两个友元函数,重载了减法和除法运算符。在主函数中,创建了两个复数对象c1和c2,以及一个空对象c3,然后对c1进行除以5的操作,将结果赋值给c3,并输出结果。但是程序出现了错误,提示没有找到接受“int”类型的右操作数的运算符,需要检查代码中的数据类型和运算符是否匹配。
相关问题
#include <iostream> #include<algorithm> #include<cmath> #include <queue> using namespace std;
#include <iostream>:这是C++标准库中的头文件,用于输入输出流操作,包括cin、cout等。
#include<algorithm>:这是C++标准库中的头文件,用于提供各种算法操作,如排序、查找等。
#include<cmath>:这是C++标准库中的头文件,用于提供数学函数操作,如绝对值、平方根等。
#include <queue>:这是C++标准库中的头文件,用于提供队列操作,包括入队、出队等。
using namespace std;:这是C++的命名空间声明,表示使用std命名空间,可以直接使用std中的函数和对象。
#include<iostream>using namespace std;
这是一个C++的代码片段,它包含了iostream头文件和使用了命名空间std。iostream头文件提供了输入输出流的功能,而using namespace std;语句则是为了方便使用std命名空间中的函数和对象,避免每次都需要写std::。
以下是一个简单的示例代码,展示了如何使用iostream头文件和命名空间std来输出一句话:
```cpp
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
```
这段代码会输出"Hello, World!",endl是一个特殊的控制符,用于在输出流中插入一个换行符。
相关推荐
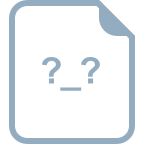
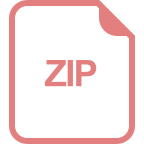













